How to Generate Unique Deterministic Integers from Another Integer?
Generating Unique Deterministic Integers from Another Integer
In the quest to create adeterministic number generation function, our aim is to construct a function where each input number generates a unique corresponding number without any duplicates.
Modular Arithmetic Solution:
The ingenious solution lies in modular arithmetic, particularly the Affine cipher. It employs the transformation formula:
f(P) = (mP + s) mod n
where:
- n represents the range of the allowed integer values (e.g., 2^64 for uint64)
- s is an arbitrary shift value less than the range
- m is a coprime (no common factors) with n
For the uint64 range, a non-even value for m is suggested to avoid divisibility by 2.
Example Implementation:
import ( "fmt" ) func main() { m := uint64(39293) s := uint64(75321908) transform := func(p uint64) uint64 { return p * m + s } testValues := []uint64{1, 2, 3, 4, 5} for _, v := range testValues { fmt.Printf("%v -> %v\n", v, transform(v)) } }
This function ensures that for all possible uint64 input values, the generated transformed values are unique.
Adapting for Signed Integers:
For signed integers (int64), the approach remains similar. We convert inputs and outputs between uint64 and int64 to maintain unique mappings:
func signedTransform(p int64) int64 { return int64(transform(uint64(p))) }
By utilizing this deterministic function, developers can generate unique and reproducible numbers from any given input integer, making it an invaluable tool for various applications.
The above is the detailed content of How to Generate Unique Deterministic Integers from Another Integer?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










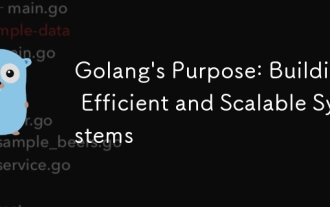
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
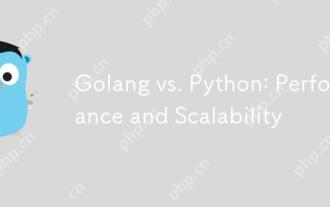
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
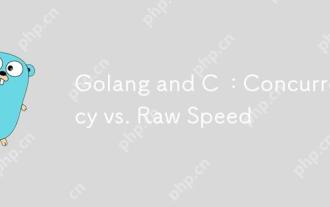
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
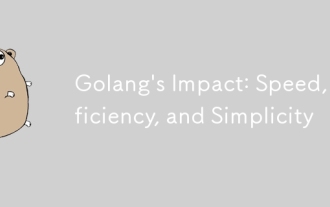
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
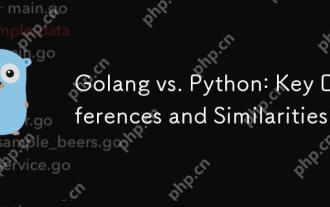
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
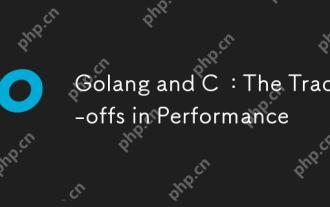
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
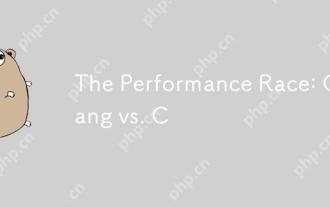
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
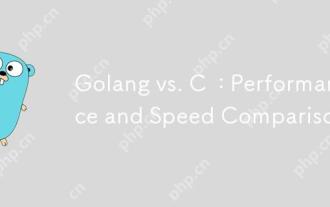
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
