How to Send HTTP POST Data Directly from PHP Code?
HTTP POST Data Handling in PHP
When sending form data to a web server, the common approach involves using an HTML form that submits the data via HTTP POST. However, in certain scenarios, you may need to send POST data directly from PHP code without relying on a form.
Direct POST Data Submission
To transmit POST data without a form in PHP, a method known as cURL (Client URL Library) can be employed. Here's how you can do it:
$url = 'http://www.example.com'; $postData = [ 'myVar1' => 'value1', 'myVar2' => 'value2' ]; $postFields = http_build_query($postData); $curl = curl_init($url); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $postFields); curl_setopt($curl, CURLOPT_FOLLOWLOCATION, true); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($curl); curl_close($curl);
In this example, we define a URL, $url, and populate a PHP array, $postData, with the key-value pairs we want to send. Using http_build_query(), we convert the array into an HTTP-formatted query string, which is then assigned to $postFields.
We initialize the curl handle and set its CURLOPT_POST option to true to indicate that we're sending POST data. The CURLOPT_POSTFIELDS option specifies the data to be sent. We also set various other options, such as CURLOPT_FOLLOWLOCATION (which follows redirects), CURLOPT_HEADER (which suppresses HTTP headers from the response), and CURLOPT_RETURNTRANSFER (which makes curl_exec() return the response).
Finally, we execute the curl request with curl_exec() and store the response in the $response variable. This response can then be parsed or processed as per your requirements.
The above is the detailed content of How to Send HTTP POST Data Directly from PHP Code?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
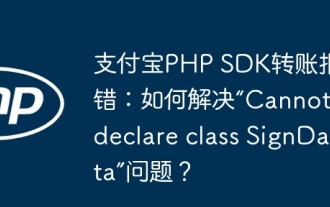
Alipay PHP...
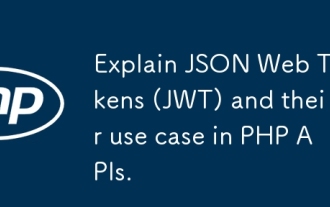
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
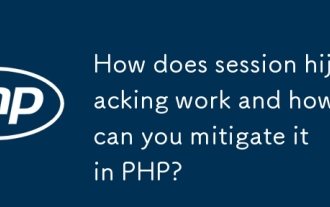
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
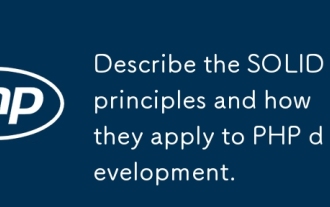
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
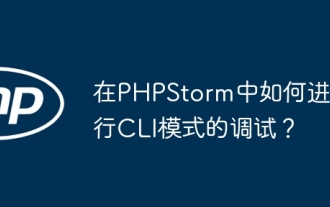
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
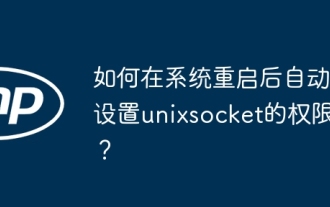
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
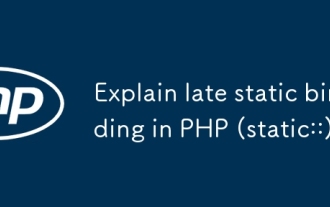
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
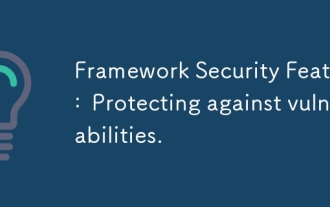
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
