


Is there an equivalent to JavaScript's `eval()` function for dynamic evaluation of Go code?
Evaluating Go Code with eval()
Introduction
In JavaScript, the eval() function allows you to execute code or expressions dynamically. Is there an equivalent function in Go that can evaluate Go code?
Answer
Yes, it is possible to evaluate Go expressions in a dynamic manner. The key component for this is the go/types package. Here's how you can do it:
Creating a Package and Scope
First, create a package object to hold the code to be evaluated, and a scope.Scope object to define the scope in which the code will execute.
package eval import ( "go/ast" "go/constant" "go/parser" "go/token" "go/types" ) var ( // Create a new package object to hold the evaluated code. pkg = types.NewPackage("eval", "example.com/eval") // Create a new scope object to define the scope of evaluation. scope = types.NewScope(nil, token.NewFileSet()) )
Inserting Constants into Scope
The eval() function typically allows evaluating expressions that reference defined variables or constants. To simulate this behavior in Go, we can insert constants into the scope of evaluation.
// Insert a constant named "x" with value 10 into the scope. scope.Insert(scope.Lookup("x"), &types.Const{ Val: constant.MakeInt64(10), Type: pkg.Scope().Lookup("int").Type(), // Lookup the "int" type from the package's scope. Pkg: pkg, Name: "x", Kind: types.Const, Obj: nil, // We don't need an Object for introducing constants directly. Alias: false, })
Parsing and Evaluating Expressions
Next, you need to parse the Go expression to be evaluated and create an AST (Abstract Syntax Tree) for it. Once you have the AST, you can evaluate the expression with the help of the go/types package.
// Parse the input Go expression. expr, err := parser.ParseExpr("x + 17") if err != nil { panic(err) } // Evaluate the expression in the defined scope. result, err := types.Eval(expr, scope) if err != nil { panic(err) }
Result and Conclusion
The result of the evaluation will be stored in the result variable as a constant.Value. You can convert it to the desired type as needed. In your example, you can obtain the result using:
intResult, ok := constant.Int64Val(result) if !ok { panic("failed to convert result to int") }
By following these steps, you can achieve dynamic evaluation of Go code, similar to the eval() function in JavaScript.
The above is the detailed content of Is there an equivalent to JavaScript's `eval()` function for dynamic evaluation of Go code?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
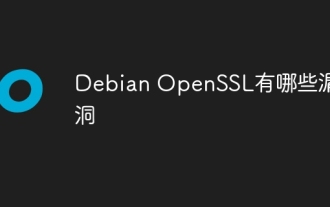
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
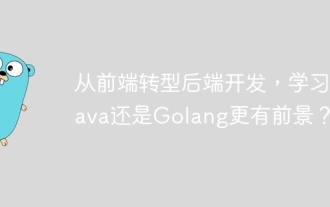
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
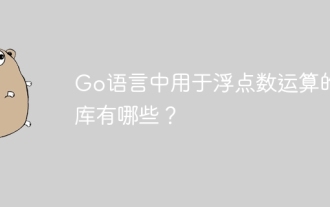
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
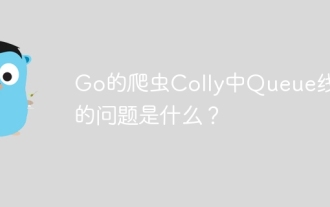
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
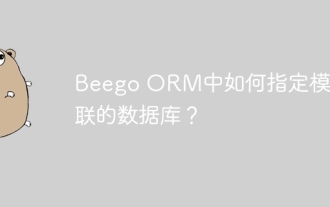
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
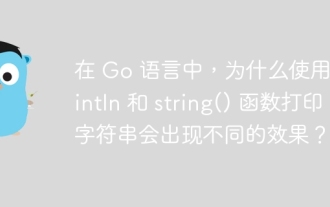
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
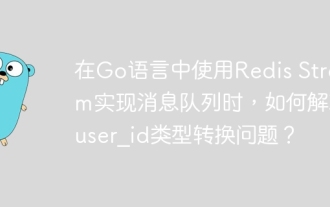
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
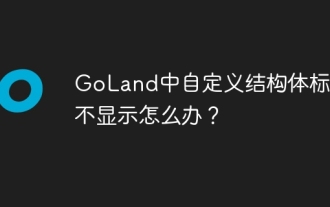
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
