


How to Reshape a Pandas DataFrame Using the Melt Function and a Dictionary?
Pandas Melt Function: Reshaping Dataframes for Analysis
Question:
Consider a dataframe with multiple columns and a dictionary:
df = pd.DataFrame([[2, 4, 7, 8, 1, 3, 2013], [9, 2, 4, 5, 5, 6, 2014]], columns=['Amy', 'Bob', 'Carl', 'Chris', 'Ben', 'Other', 'Year'])<br>
d = {'A': ['Amy'], 'B': ['Bob', 'Ben'], 'C': ['Carl', 'Chris']}<br>
How do we reshape the dataframe to resemble the following structure, where columns are melted and grouped?
Group Name Year Value<br> 0 A Amy 2013 2<br> 1 A Amy 2014 9<br> 2 B Bob 2013 4<br> 3 B Bob 2014 2<br> 4 B Ben 2013 1<br> 5 B Ben 2014 5<br> 6 C Carl 2013 7<br> 7 C Carl 2014 4<br> 8 C Chris 2013 8<br> 9 C Chris 2014 5<br>10 Other 2013 3<br>11 Other 2014 6<br>
Answer:
To reshape the dataframe using the melt function, follow these steps:
-
Melt the dataframe: Melt the dataframe into a wide format using the melt function. This will convert the columns into rows, with the id_vars parameter used to specify the columns that should remain intact.
m = pd.melt(df, id_vars=['Year'], var_name='Name')
Copy after login -
Create a mapping dictionary: Reshape the dictionary d to create a mapping between column names and group names.
d2 = {} for k, v in d.items(): for item in v: d2[item] = k
Copy after login -
Add 'Group': Map the newly created dictionary d2 to the 'Name' column to add the 'Group' column.
m['Group'] = m['Name'].map(d2)
Copy after login -
Move 'Other': Move 'Other' values from the 'Name' column to the 'Group' column.
mask = m['Name'] == 'Other' m.loc[mask, 'Name'] = '' m.loc[mask, 'Group'] = 'Other'
Copy after login
The resulting dataframe will contain the desired flattened structure:
print(m) Year Name value Group 0 2013 Amy 2 A 1 2014 Amy 9 A 2 2013 Bob 4 B 3 2014 Bob 2 B 4 2013 Carl 7 C ... ... ... ... ... 7 2014 Chris 5 C 8 2013 Ben 1 B 9 2014 Ben 5 B 10 2013 3 Other 11 2014 6 Other
The above is the detailed content of How to Reshape a Pandas DataFrame Using the Melt Function and a Dictionary?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










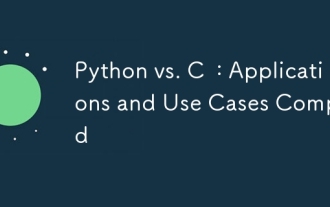
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
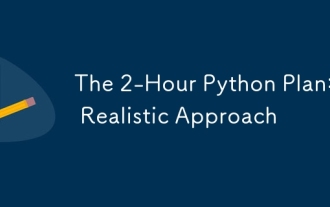
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
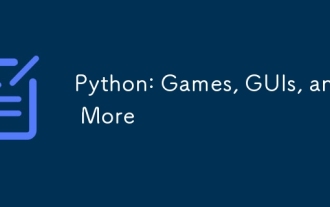
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
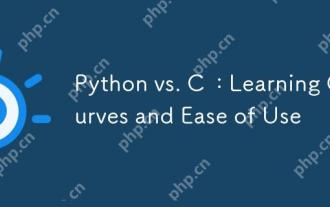
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
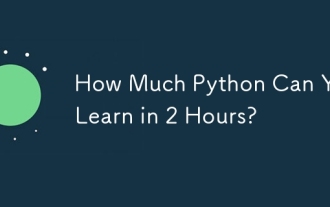
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
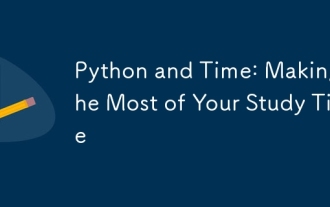
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
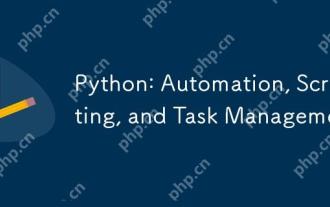
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
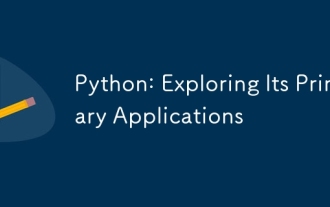
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
