How Can Python Functions Return Multiple Values?
Returning Multiple Values from Python Functions
In Python, a function can only return a single value. However, there are several ways to effectively return multiple values from a function.
Returning a Tuple
Tuples are immutable sequences that can hold any number of values. To return a tuple from a function, simply use the return statement followed by the values enclosed in parentheses or separated by commas. For example:
def get_coordinates(): x = input("Enter the x-coordinate: ") y = input("Enter the y-coordinate: ") return x, y
When called, this function will return a tuple containing the x and y coordinates. You can assign these values to separate variables as follows:
x, y = get_coordinates()
Returning a List
Lists are mutable sequences that can also be used to return multiple values from a function. The syntax is similar to returning a tuple, but the values are enclosed in square brackets.
def get_stats(): mean = calculate_mean() median = calculate_median() return [mean, median]
To assign the returned values to separate variables, use the following syntax:
mean, median = get_stats()
Using a Custom Class or NamedTuple
If you need to return multiple values that are related, you can create a custom class or use a NamedTuple to encapsulate the data. This approach can improve code readability and maintainability.
from dataclasses import dataclass @dataclass class Coordinates: x: float y: float def get_coordinates(): x = input("Enter the x-coordinate: ") y = input("Enter the y-coordinate: ") return Coordinates(x, y) coordinates = get_coordinates() print(f"X-coordinate: {coordinates.x}, Y-coordinate: {coordinates.y}")
Conclusion
There are several ways to return multiple values from a Python function. The best approach depends on the specific use case and desired data structure. Tuples and lists are simple and convenient options, while custom classes and NamedTuples offer more flexibility and code organization.
The above is the detailed content of How Can Python Functions Return Multiple Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










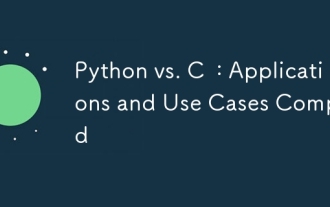
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
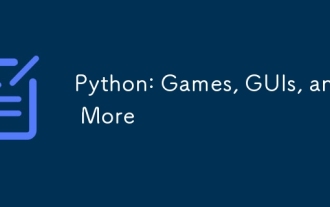
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
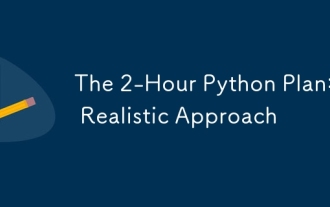
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
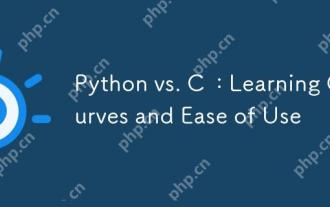
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
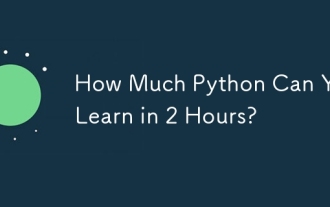
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
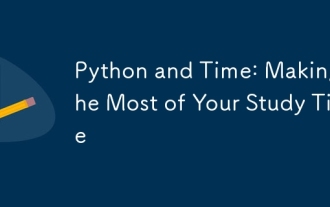
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
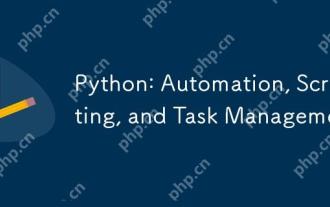
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
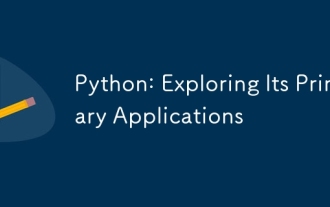
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
