


How to Specify Function Types in Type Hints with `typing.Callable`?
Specifying Function Types in Type Hints
In type hints, specifying the type of a variable as a function might seem challenging without a dedicated "typing.Function" class or guidance in PEP 483. However, the solution lies in utilizing "typing.Callable."
By leveraging "typing.Callable," you can declare function types in your annotations. For instance:
from typing import Callable def my_function(func: Callable):
It's important to note that "Callable" by itself is equivalent to "Callable[..., Any]." This implies that the callable function accepts any number and type of arguments (...) and returns a value of any type (Any).
If you require stricter constraints, you can specify the input argument types and return type explicitly. For example, consider the function "sum":
def sum(a: int, b: int) -> int: return a+b
Its corresponding annotation would be:
Callable[[int, int], int]
In this annotation, the parameters are specified within the square brackets, and the return type is specified as the second element within the square brackets. The syntax for specifying function types in general is:
Callable[[ParamType1, ParamType2, ..., ParamTypeN], ReturnType]
The above is the detailed content of How to Specify Function Types in Type Hints with `typing.Callable`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
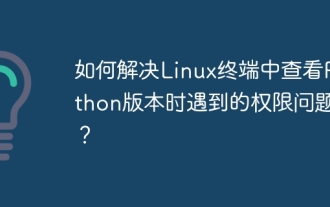
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
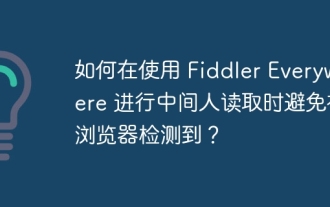
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
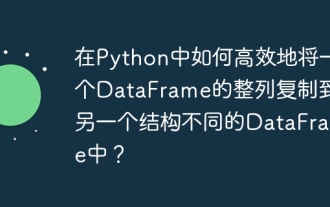
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
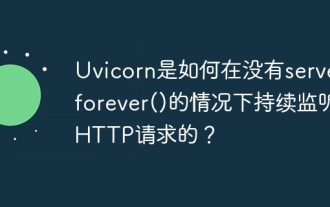
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
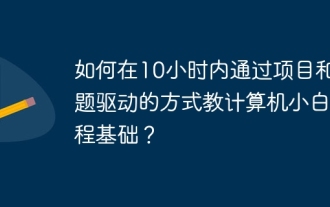
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
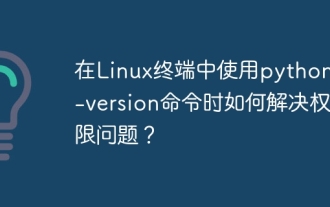
Using python in Linux terminal...
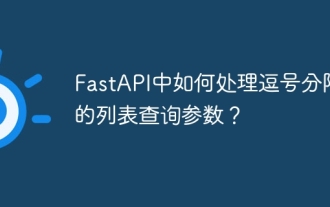
Fastapi ...
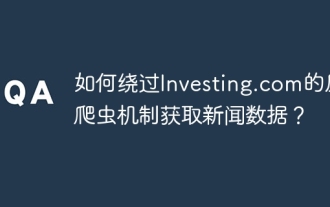
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
