


How can I use Python to query the Google Maps API and parse JSON responses?
Nov 23, 2024 am 04:25 AMHTTP Requests and JSON Parsing in Python
This tutorial demonstrates how to effectively perform HTTP requests and parse JSON responses in Python, specifically tailored for querying the Google Maps API.
Problem:
Our goal is to dynamically query the Google Maps API via the Google Directions API. For instance, consider the request below:
http://maps.googleapis.com/maps/api/directions/json?origin=Chicago,IL&destination=Los+Angeles,CA&waypoints=Joplin,MO|Oklahoma+City,OK&sensor=false
This request fetches driving directions from Chicago, IL to Los Angeles, CA, with waypoints in Joplin, MO and Oklahoma City, OK. The response is returned in JSON format.
Solution:
To implement this in Python, we utilize the popular requests library:
import requests url = 'http://maps.googleapis.com/maps/api/directions/json' params = { 'origin': 'Chicago,IL', 'destination': 'Los+Angeles,CA', 'waypoints': 'Joplin,MO|Oklahoma+City,OK', 'sensor': 'false' } resp = requests.get(url, params=params) data = resp.json() # Converts the JSON response into a dictionary
The requests library handles the HTTP request and provides the response in a convenient format. The resp object contains the response status code and headers, while the data variable is a dictionary containing the parsed JSON response.
For further information on understanding JSON response content, please refer to the documentation provided by the requests library.
The above is the detailed content of How can I use Python to query the Google Maps API and parse JSON responses?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
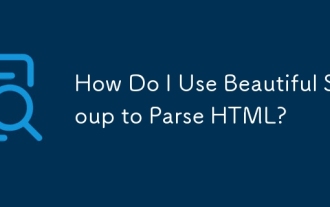
How Do I Use Beautiful Soup to Parse HTML?
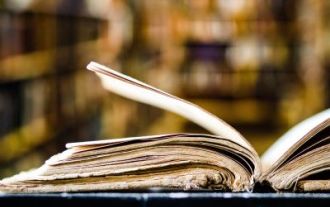
How to Use Python to Find the Zipf Distribution of a Text File
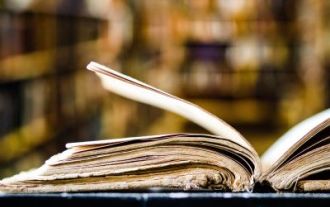
How to Work With PDF Documents Using Python
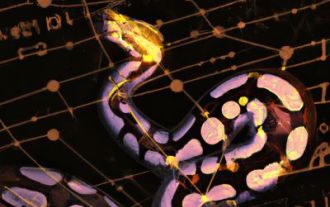
How to Cache Using Redis in Django Applications
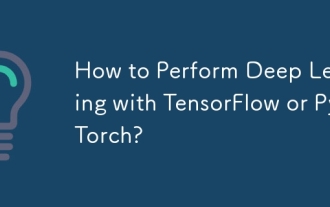
How to Perform Deep Learning with TensorFlow or PyTorch?
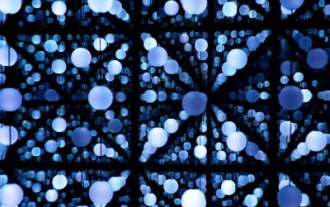
How to Implement Your Own Data Structure in Python
