How Can I Rename a Function in C ?
Renaming Functions in C
Assigning a new name to a function is not as straightforward as it is for types, variables, or namespaces. However, there are several approaches available:
1. Macros:
The simplest method is to use a macro definition. For example, #define holler printf. This creates a symbol alias, but it's a preprocessor directive and not a true function alias.
2. Function Pointers and References:
Function pointers and references can refer to an existing function. For instance, void (*p)() = fn; creates a pointer that points to fn, and void (&&r)() = fn; creates a reference to fn.
3. Inline Function Wrappers:
Inline function wrappers are another option. You can define a new function that simply calls the original function. For example, inline void g(){ f(); }.
4. Alias Templates (C 11 and Later):
With C 11, you can use alias templates for non-template, non-overloaded functions:
const auto& new_fn_name = old_fn_name;
For overloaded functions, use a static cast:
const auto& new_fn_name = static_cast<OVERLOADED_FN_TYPE>(old_fn_name);
5. Constexpr Template Variables (C 14 and Later):
C 14 introduced constexpr template variables, which enable aliasing of templated functions:
template<typename T> constexpr void old_function(/* args */); template<typename T> constexpr auto alias_to_old = old_function<T>;
6. std::mem_fn (C 11 and Later):
For member functions, you can use the std::mem_fn function to create an alias:
auto greet = std::mem_fn(&A::f);
The above is the detailed content of How Can I Rename a Function in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


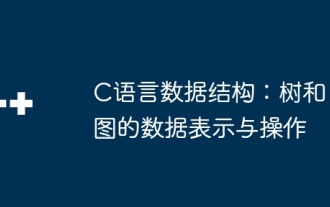
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
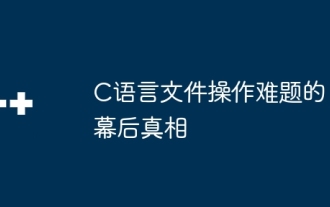
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
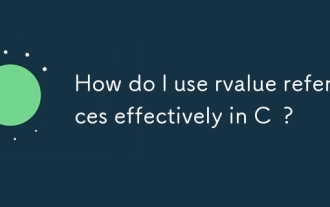
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
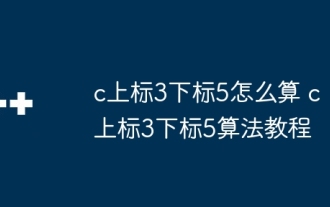
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
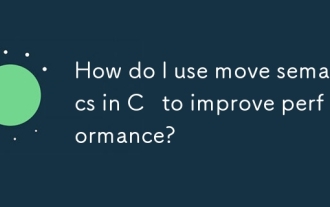
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
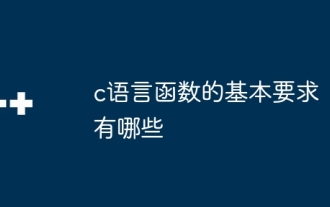
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
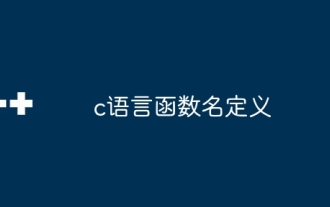
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
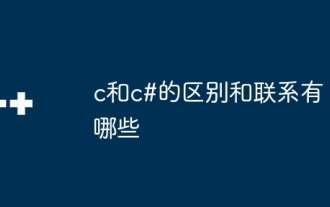
Although C and C# have similarities, they are completely different: C is a process-oriented, manual memory management, and platform-dependent language used for system programming; C# is an object-oriented, garbage collection, and platform-independent language used for desktop, web application and game development.
