How to Allow Non-Continuous Cell Selection in a JTable?
Allowing Individual and Non-Continuous JTable Cell Selection
Understanding the Requirement
The task is to enable users to select multiple non-contiguous cells in a JTable, allowing for more flexible cell selection beyond groups of continuous cells.
Exploring Solutions
1. Utilizing ListSelectionModel
The JTable's setSelectionModel() method allows you to customize the selection model. By setting it to ListSelectionModel.SINGLE_SELECTION and adding a listener to handle InputEvent.CTRL_MASK, you can implement non-continuous cell selection.
2. Customizing MouseEvent Processing
By overriding the processMouseEvent() method in the JTable, you can modify the event's modifiers to indicate that the control key is pressed. This way, the table will behave as if the user is holding down the control key, enabling multiple cell selection.
3. Implementing a Custom List Selection Model
If the built-in selection models don't meet your requirements, you can create your own ListSelectionModel implementation that manages cell selection in the desired manner.
4. Example Implementation
The following code snippet provides an SSCCE that demonstrates the custom processMouseEvent() approach for allowing non-continuous cell selection in a JTable:
import java.awt.Component; import java.awt.event.InputEvent; import java.awt.event.MouseEvent; import javax.swing.*; public class TableSelection extends JFrame { public TableSelection() { JPanel main = new JPanel(); // Initialize table data String[] columnNames = {"First Name", "Last Name", "Sport", "# of Years", "Vegetarian"}; Object[][] data = { {"Kathy", "Smith", "Snowboarding", new Integer(5), new Boolean(false)}, {"John", "Doe", "Rowing", new Integer(3), new Boolean(true)}, {"Sue", "Black", "Knitting", new Integer(2), new Boolean(false)}, {"Jane", "White", "Speed reading", new Integer(20), new Boolean(true)}, {"Joe", "Brown", "Pool", new Integer(10), new Boolean(false)} }; // Override processMouseEvent to add control modifier JTable table = new JTable(data, columnNames) { @Override protected void processMouseEvent(MouseEvent e) { int modifiers = e.getModifiers() | InputEvent.CTRL_MASK; MouseEvent myME = new MouseEvent((Component) e.getSource(), e.getID(), e.getWhen(), modifiers, e.getX(), e.getY(), e.getXOnScreen(), e.getYOnScreen(), e.getClickCount(), e.isPopupTrigger(), e.getButton()); super.processMouseEvent(myME); } }; JScrollPane pane = new JScrollPane(table); main.add(pane); this.add(main); } public static void main(String[] args) { new TableSelection(); } }
This implementation allows you to select non-contiguous cells by holding down the control key while clicking on the desired cells.
The above is the detailed content of How to Allow Non-Continuous Cell Selection in a JTable?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










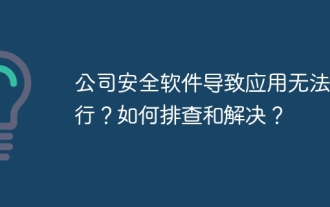
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
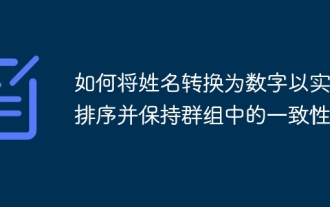
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
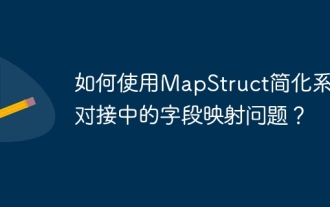
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
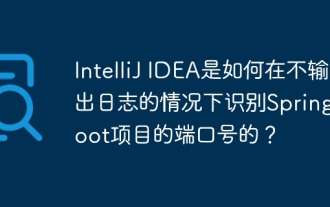
Start Spring using IntelliJIDEAUltimate version...
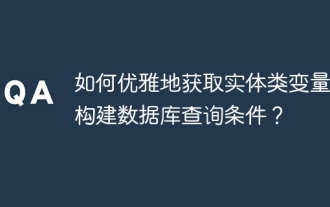
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
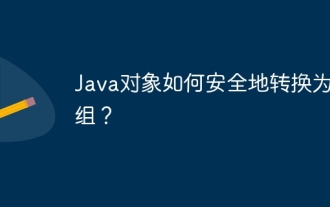
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
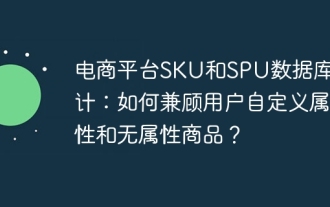
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
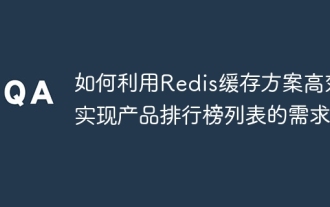
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
