


How Can a Go Multiplexer Ensure Fair and Safe Access Across Multiple Input Channels?
A Channel Multiplexer with Equal Access
This Go multiplexer aims to merge the outputs of multiple channels into one, ensuring that each input channel has equal rights to the output channel. However, the provided test gives unexpected results.
Analysis of the Issue
The critical issue lies in the goroutines spawned from the Mux function. The channel parameter c, intended to represent each input channel, is being updated on each iteration of the loop. This means that all goroutines end up pulling from the same channel instead of their intended individual channels.
Solution
To resolve this issue, modify the goroutine creation loop to pass the correct channel to each goroutine:
for _, c := range channels { go func(c <-chan big.Int) { ... }(c) }
By doing this, each goroutine captures the value of the channel when it is created, eliminating the issue and producing the desired results.
Improving Concurrency Safety
In addition to ensuring equal access to the output channel, it is crucial to ensure concurrency safety. The initial code uses an int variable n to track the closing of input channels. However, with GOMAXPROCS greater than 1, it is possible for multiple goroutines to access n simultaneously, potentially causing race conditions.
A safer approach is to use a sync.WaitGroup object, which allows goroutines to wait for each other and ensures that n is updated safely. The revised code using a sync.WaitGroup:
import ( "math/big" "sync" ) // ... other code ... // The channel to output to. ch := make(chan big.Int, len(channels)) var wg sync.WaitGroup wg.Add(len(channels)) // ... other code ... // Close the channel when the pumping is finished. go func() { // Wait for everyone to be done. wg.Wait() // Close. close(ch) }()
With these modifications, the multiplexer now operates correctly and safely, ensuring that all input channels have equal access to the output channel and avoiding race conditions.
The above is the detailed content of How Can a Go Multiplexer Ensure Fair and Safe Access Across Multiple Input Channels?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










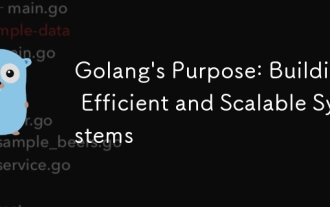
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
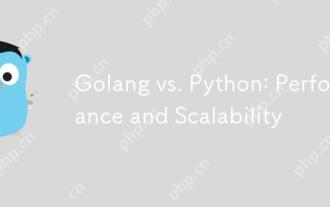
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
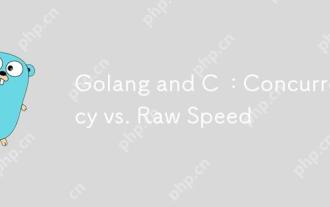
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
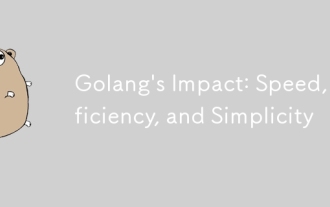
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
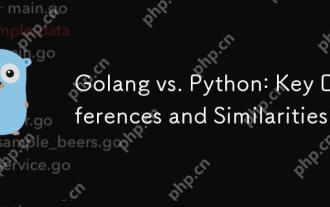
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
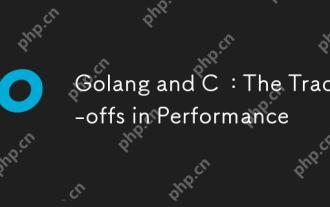
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
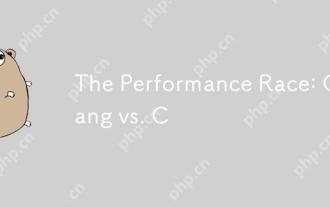
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
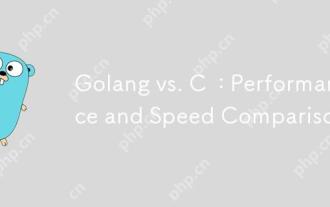
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
