How to Escape Go Strings in Regular Expressions?
Escaping Go Strings in Regular Expressions
Matching strings with special characters like periods, dashes, or other unique elements can be challenging in regular expressions. This dilemma arises when the string needs to be escaped to prevent conflicts with regex syntax.
Introducing regexp.QuoteMeta
In Go, there is a built-in function named regexp.QuoteMeta that provides a solution for this problem.
Consider the following case, where you want to match a string defined as {{string}} in a regular expression that starts with ^(@|s)*{{string}}:?. The original {{string}} may contain periods or dashes, which can conflict with regex syntax.
Using regexp.QuoteMeta for String Escaping
To overcome this challenge, you can use regexp.QuoteMeta as follows:
import ( "regexp" ) // define the string to be escaped stringToEscape := "{{string}}" // escape the string using regexp.QuoteMeta escapedString := regexp.QuoteMeta(stringToEscape) // create a regular expression using the escaped string r := regexp.MustCompile("^(@|\s)*" + escapedString + ":?$")
By using regexp.QuoteMeta, you can ensure that the special characters in stringToEscape are escaped properly, enabling you to match the string effectively within the regular expression. This function is a convenient tool for working with strings in regex expressions.
The above is the detailed content of How to Escape Go Strings in Regular Expressions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




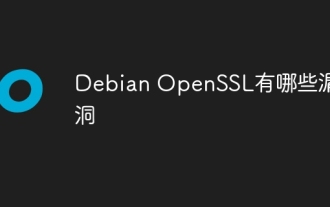
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
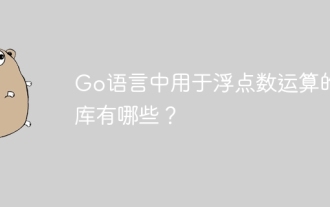
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
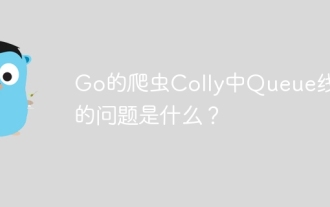
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
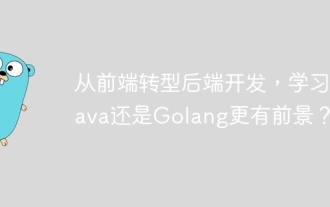
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
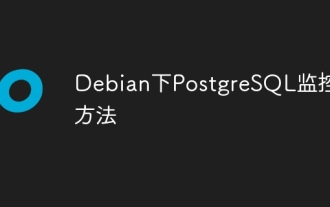
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
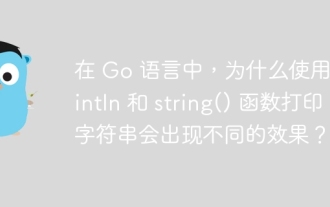
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
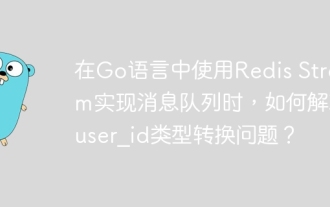
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
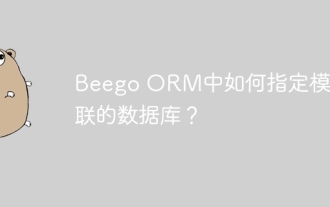
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
