How Can I Create a File Instance from a Resource Inside a JAR File?
Creating a File Instance from a Resource in a Jar
Creating a File instance from a resource retrieved from a JAR file can be tricky, but there are ways to achieve it.
Solution using java.io.File:
The provided code snippet demonstrates how to create a File instance from a URL retrieved using the classloader:
URL dir_url = ClassLoader.getSystemResource(dir_path); File dir = new File(dir_url.toURI());
This allows you to manipulate the files from the JAR as if they were located in the file system.
Alternative Approach Using ClassLoader:
To avoid using java.io.File altogether, you can load a directory from the classpath and list its contents directly using the classloader:
URL dir_url = ClassLoader.getSystemResource(dir_path); Enumeration<URL> files = dir_url.openStream().available(); while (files.hasMoreElements()) { // Process each file or entity }
Loading a Stream from the Resource:
To load a file as a stream, you can use the following code:
InputStream stream = ClassLoader.getSystemResourceAsStream(dir_path + "/" + file);
This provides you with a stream from which you can read the file's content.
The above is the detailed content of How Can I Create a File Instance from a Resource Inside a JAR File?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










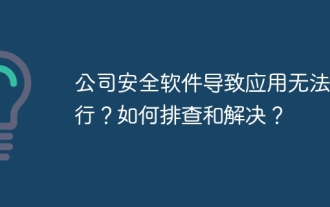
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
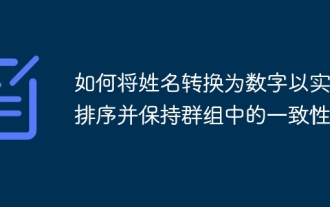
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
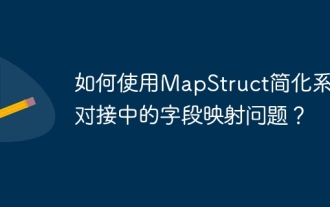
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
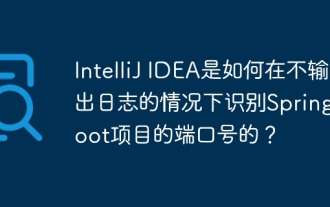
Start Spring using IntelliJIDEAUltimate version...
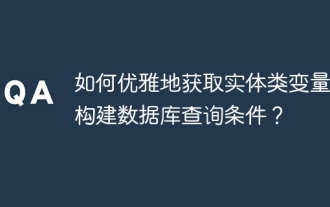
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
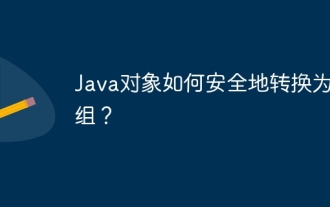
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
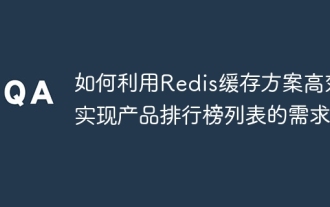
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
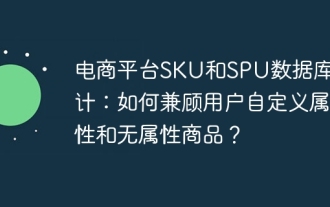
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
