


How Can I Package a Java Application with Third-Party JAR Files and DLL Dependencies into a Single JAR?
Packaging Third-Party JAR Files with DLL Dependencies
Q: I have a Java library that requires two DLL files and my own Java program that utilizes this library. How can I combine all components into a single JAR file that includes both my code, the third-party JAR, and the DLLs?
A: To create a JAR file that incorporates DLL dependencies:
- Package the DLLs: Include the DLL files anywhere within the JAR structure.
- Extract the DLLs: Before using the DLLs, extract them from the JAR to a specified location on your hard drive. This is essential for further processing.
In the realm of packaging, DLLs and other files are treated similarly to files in a ZIP archive. You can use the following Java code to extract DLLs from a JAR:
import java.io.*; import java.util.zip.ZipEntry; import java.util.zip.ZipFile; public class ExtractDLLs { public static void main(String[] args) { String jarPath = "path/to/my.jar"; String outputDirectory = "path/to/output"; try (ZipFile jarFile = new ZipFile(jarPath)) { Enumeration<? extends ZipEntry> entries = jarFile.entries(); while (entries.hasMoreElements()) { ZipEntry entry = entries.nextElement(); if (entry.isDirectory()) { continue; } if (entry.getName().endsWith(".dll")) { InputStream in = jarFile.getInputStream(entry); OutputStream out = new FileOutputStream(new File(outputDirectory, entry.getName())); byte[] buffer = new byte[1024]; int len; while ((len = in.read(buffer)) > 0) { out.write(buffer, 0, len); } in.close(); out.close(); } } } catch (IOException e) { e.printStackTrace(); } } }
Remember, packaging DLLs or other files into a JAR is as simple as packaging files into a ZIP archive. The provided code demonstration utilizes a ZIP file structure to extract DLLs from a JAR.
The above is the detailed content of How Can I Package a Java Application with Third-Party JAR Files and DLL Dependencies into a Single JAR?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










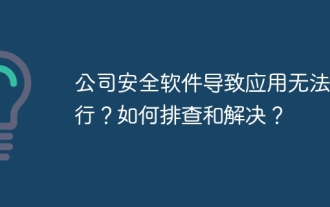
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
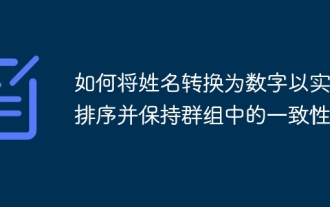
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
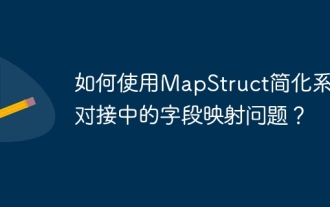
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
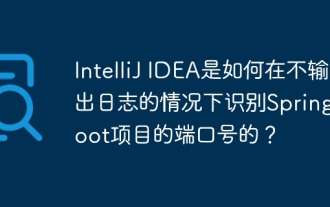
Start Spring using IntelliJIDEAUltimate version...
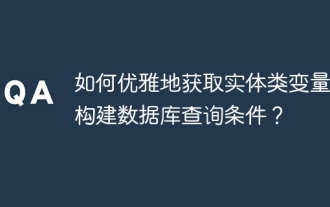
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
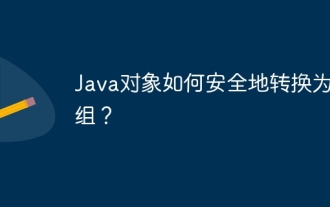
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
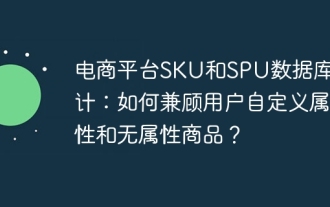
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
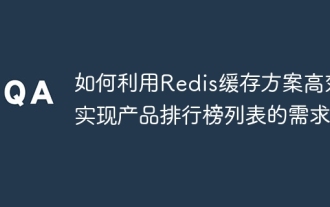
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
