How to Write PHP Form Data to a TXT File?
How to Write Form Input to a TXT File with PHP
Are you trying to capture form input and save it to a TXT file using PHP? If you've encountered difficulties and a blank page, here's a detailed solution:
1. Form Structure:
The HTML form should have the correct "method" attribute:
<form action="myprocessingscript.php" method="POST"> <input name="field1" type="text" /> <input name="field2" type="text" /> <input type="submit" name="submit" value="Save Data" /> </form>
2. PHP Script:
The PHP script uses the file_put_contents function to write to a TXT file:
<?php if (isset($_POST['field1']) && isset($_POST['field2'])) { $data = $_POST['field1'] . '-' . $_POST['field2'] . "\r\n"; $ret = file_put_contents('/tmp/mydata.txt', $data, FILE_APPEND | LOCK_EX); if ($ret === false) { die('There was an error writing this file'); } else { echo "$ret bytes written to file"; } } else { die('no post data to process'); }
3. File Permissions:
Ensure that the PHP script has write permissions to the target directory, such as /tmp in the example.
4. Alternative Approach:
You can also use the fopen() , fwrite() , and fclose() functions:
<?php $txt = "data.txt"; $fh = fopen($txt, 'w+'); if (isset($_POST['field1']) && isset($_POST['field2'])) { $txt=$_POST['field1'].' - '.$_POST['field2']; fwrite($fh,$txt."\n"); } fclose($fh);
5. Additional Notes:
- We wrote to /tmp/mydata.txt because the current working directory in your example is unknown.
- file_put_contents is a preferred approach due to its simplicity.
- For further information, refer to the file_put_contents documentation.
The above is the detailed content of How to Write PHP Form Data to a TXT File?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


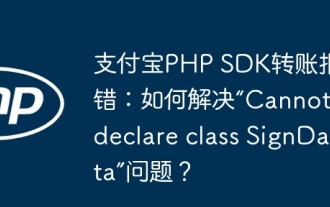
Alipay PHP...
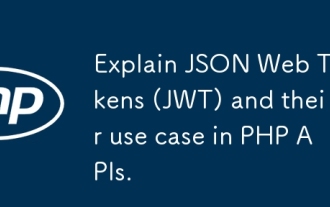
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
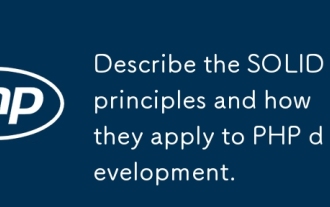
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
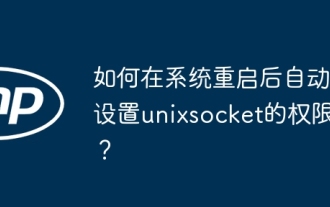
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
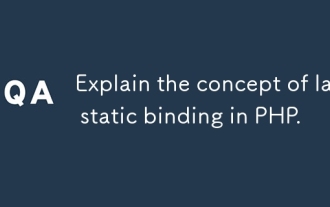
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
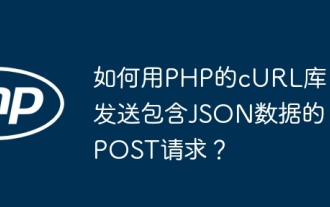
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
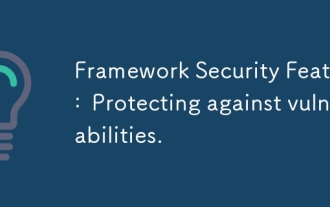
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
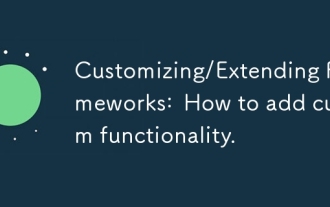
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
