How to Left-Pad Strings with Zeros in Java?
Left Zero-Padding Strings: A Comprehensive Solution
Left zero-padding strings is a common task in various programming contexts. This article explores a solution to left-pad a string with zeros using the String.format() function.
Left Padding Numeric Strings with Zeros
For strings that consist solely of numbers, a straightforward approach is to convert them to integers and then apply padding:
int num = Integer.parseInt("129018"); String padded = String.format("%010d", num); System.out.println(padded); // Output: "0000129018"
Zero-Padding Non-Numeric Strings
If the string contains non-numeric characters, the above method will not work. In such cases, a more general approach is required:
StringBuilder sb = new StringBuilder(); int numZeros = 10 - "mystring".length(); for (int i = 0; i < numZeros; i++) { sb.append('0'); } sb.append("mystring"); String padded = sb.toString(); System.out.println(padded);
This approach manually constructs a string by appending the desired number of zeros, followed by the original string.
Note: The total output length, as specified in the question (10), can be customized by adjusting the numZeros variable.
The above is the detailed content of How to Left-Pad Strings with Zeros in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




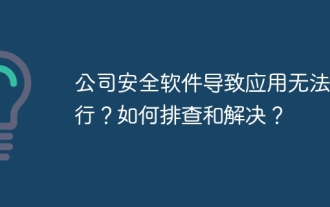
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
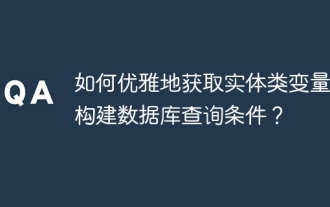
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
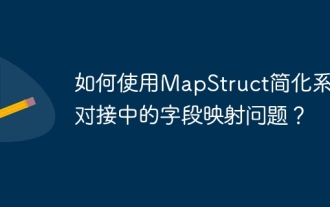
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
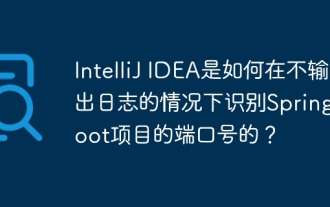
Start Spring using IntelliJIDEAUltimate version...
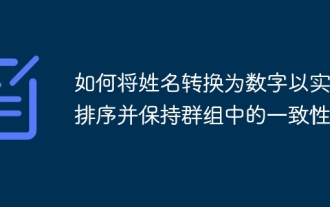
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
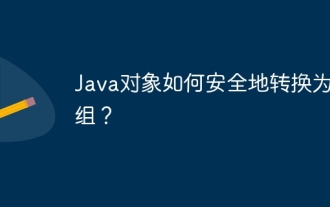
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
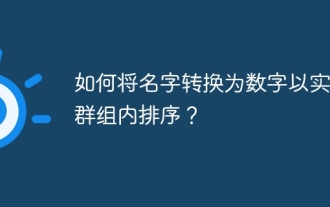
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
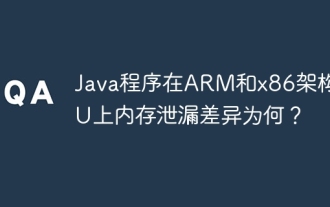
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
