My React Journey: Day 5
Today, I ventured into the world of DOM Manipulation, a fundamental concept in web development. The Document Object Model (DOM) is the bridge between HTML and JavaScript, allowing us to dynamically interact with and modify web pages. Here's what I learned:
What is DOM Manipulation?
DOM Manipulation is the process of using JavaScript to interact with and change the structure, style, or content of a webpage.
Accessing Elements
To manipulate the DOM, we first need to select or access the elements. JavaScript provides several methods to achieve this:
getElementById: Selects an element by its ID.
const header = document.getElementById('main-header'); console.log(header); // Logs the element with ID 'main-header'
querySelector: Selects the first matching element using CSS selectors.
const firstButton = document.querySelector('.btn'); console.log(firstButton); // Logs the first element with class 'btn'
querySelectorAll: Selects all matching elements as a NodeList.
const allButtons = document.querySelectorAll('.btn'); console.log(allButtons); // Logs a list of all elements with class 'btn'
Other Methods:
- getElementsByClassName (selects elements by class name).
- getElementsByTagName (selects elements by tag name).
Manipulating Elements
1. Changing Content
Use the innerHTML or textContent property to change the content of an element.
const title = document.getElementById('title'); title.innerHTML = 'Welcome to My React Journey!'; title.textContent = 'Day 5 - DOM Manipulation';
2. Changing Styles
You can dynamically update styles using the style property.
const button = document.querySelector('.btn'); button.style.backgroundColor = 'blue'; button.style.color = 'white';
3. Adding/Removing Classes
Use the classList property to add, remove, or toggle classes.
button.classList.add('active'); // Adds 'active' class button.classList.remove('btn'); // Removes 'btn' class button.classList.toggle('hidden'); // Toggles 'hidden' class
4. Attributes
You can modify attributes like src, alt, href, etc.
const image = document.querySelector('img'); image.setAttribute('src', 'new-image.jpg'); image.setAttribute('alt', 'A beautiful scenery');
Event Handling
DOM manipulation often goes hand-in-hand with events. You can listen for user interactions like clicks, keypresses, or mouse movements.
Example: Adding a Click Event
const button = document.querySelector('.btn'); button.addEventListener('click', () => { alert('Button clicked!'); });
Example: Updating Content on Input
const input = document.querySelector('#name-input'); input.addEventListener('input', () => { const display = document.querySelector('#name-display'); display.textContent = `Hello, ${input.value}!`; });
Dynamic Element Creation
You can create and append elements dynamically.
const newElement = document.createElement('p'); newElement.textContent = 'This is a new paragraph added dynamically!'; document.body.appendChild(newElement);
Final Thoughts
DOM Manipulation is incredibly powerful, allowing developers to create interactive and dynamic web pages. It forms the foundation of frameworks like React, where DOM updates are handled more efficiently using virtual DOMs.
I’m excited to see how these concepts play out as I progress further in my React Native journey.
Day 6, here I come! ?
The above is the detailed content of My React Journey: Day 5. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
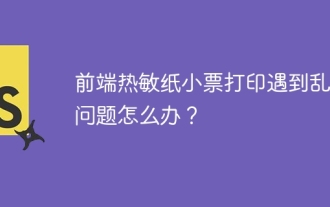
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
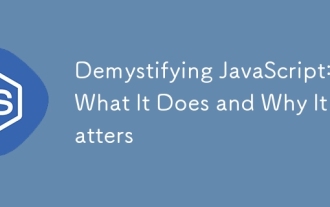
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
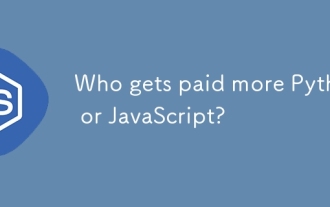
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
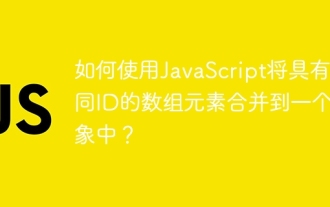
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
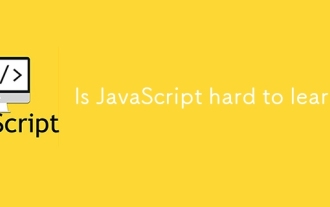
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
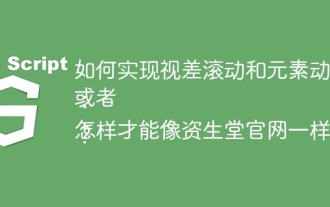
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
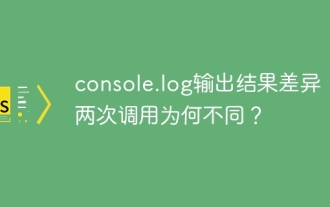
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
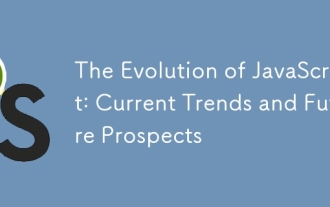
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
