How to Get Query Parameter Values from a URL Using jQuery?
How to Extract Query Parameters from URL Using jQuery
Question:
How do you obtain the value of a query parameter from a URL using jQuery and use it in your code? For instance, if the URL is http://www.mysite.co.uk/?location=mylocation1, how do you assign the "mylocation1" value to a variable for use in the following jQuery statement:
var thequerystring = "getthequerystringhere" $('html,body').animate({scrollTop: $(""div#" + thequerystring).offset().top}, 500);
Answer:
To extract query parameters from a URL, you can use the following code snippet:
// Read a page's GET URL variables and return them as an associative array. function getUrlVars() { var vars = [], hash; var hashes = window.location.href.slice(window.location.href.indexOf('?') + 1).split('&'); for(var i = 0; i < hashes.length; i++) { hash = hashes[i].split('='); vars.push(hash[0]); vars[hash[0]] = hash[1]; } return vars; }
This function takes the URL parameters from the browser's address bar, splits them into key-value pairs, and returns them as an object. For example, the URL http://www.example.com/?me=myValue&name2=SomeOtherValue would return the following object:
{ "me" : "myValue", "name2" : "SomeOtherValue" }
With this function, you can access the query parameters by their keys. In your case, to obtain the "location" parameter, you can write:
var locationValue = getUrlVars()["location"];
Finally, you can use the extracted parameter value in your jQuery statement as follows:
var thequerystring = locationValue; $('html,body').animate({scrollTop: $(""div#" + thequerystring).offset().top}, 500);
The above is the detailed content of How to Get Query Parameter Values from a URL Using jQuery?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


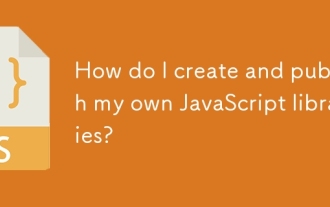
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
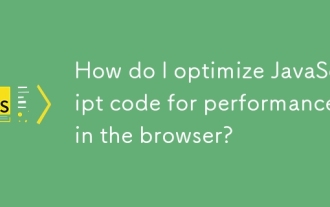
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
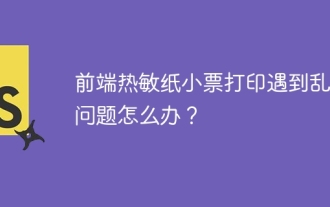
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
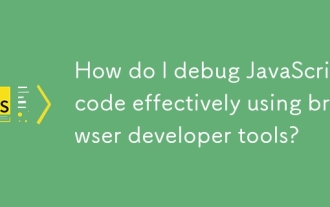
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
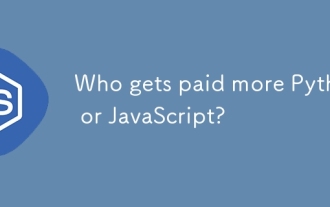
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
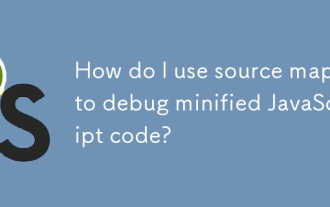
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
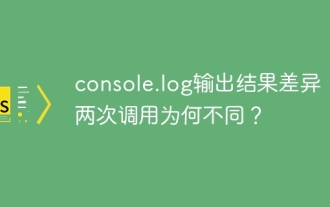
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
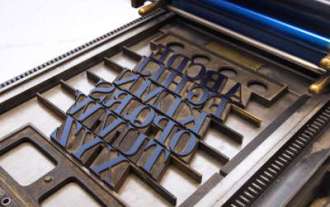
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript
