


How to Dynamically Evaluate Expressions from Formulas in Pandas using pd.eval?
Dynamically evaluate an expression from a formula in Pandas
The evaluation of arithmetic expressions on one or more dataframe columns using pd.eval is a common task, especially when automating workflows. Consider the following code snippet:
`x = 5
df2['D'] = df1['A'] (df1['B'] * x)``
This code adds a new column D to df2 by performing an operation on the columns A and B from df1, and multiplying the result by a variable x. The goal is to repeat this data manipulation dynamically, leveraging pd.eval's ability to execute expressions as strings.
First, let's introduce the input DataFrames:
import pandas as pd import numpy as np np.random.seed(0) df1 = pd.DataFrame(np.random.choice(10, (5, 4)), columns=list('ABCD')) df2 = pd.DataFrame(np.random.choice(10, (5, 4)), columns=list('ABCD')) df1 A B C D 0 5 0 3 3 1 7 9 3 5 2 2 4 7 6 3 8 8 1 6 4 7 7 8 1 df2 A B C D 0 5 9 8 9 1 4 3 0 3 2 5 0 2 3 3 8 1 3 3 4 3 7 0 1
To evaluate the expression dynamically using pd.eval, one can use the following code:
result = pd.eval('df1.A (df1.B * x)')
This line of code creates a new DataFrame called result that contains the evaluated expression. The eval function can also be used to perform conditional evaluations, such as:
pd.eval('df1.A > df2.A')
To assign the result of the expression back to df2, use the following syntax:
df2['D'] = pd.eval('df1.A (df1.B * x)', target=df2)
To pass an argument inside the expression string, use the @ symbol:
pd.eval('df1.A (df1.B * @x)', local_dict={'x': 5})
For maximum performance, consider the following arguments:
parser='python' for controlling the syntax rules and ensuring consistency with Python's operator precedence.
engine='numexpr' for faster evaluation using the optimized numexpr backend.
This should provide you with a comprehensive understanding of how to dynamically evaluate expressions from formulas in Pandas using pd.eval.
The above is the detailed content of How to Dynamically Evaluate Expressions from Formulas in Pandas using pd.eval?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










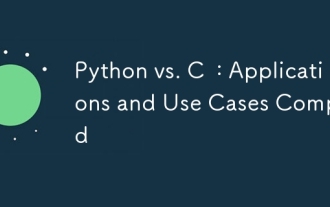
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
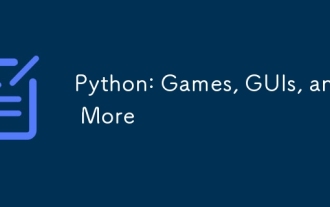
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
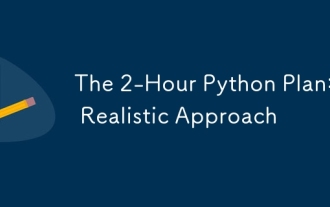
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
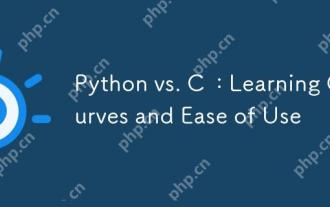
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
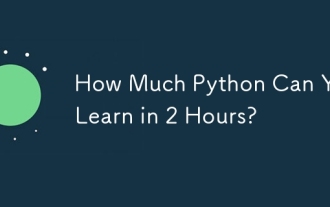
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
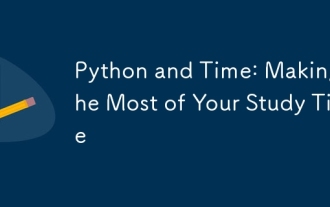
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
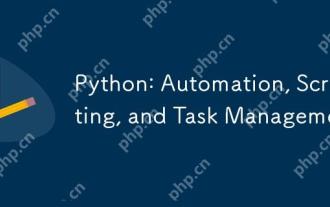
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
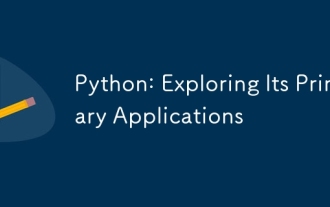
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
