


How to Connect a JavaFX Application to a MySQL Database and Display Data in a TableView?
JavaFX MySQL Database Connection
Connecting JavaFX applications to MySQL databases requires a specific class that handles the connection and data retrieval. Here's a simple example:
PersonDataAccessor:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; import java.sql.Statement; import java.sql.ResultSet; import java.util.List; import java.util.ArrayList; public class PersonDataAccessor { private Connection connection; // Constructor public PersonDataAccessor(String driverClassName, String dbURL, String user, String password) throws SQLException, ClassNotFoundException { Class.forName(driverClassName); connection = DriverManager.getConnection(dbURL, user, password); } // Close connection public void shutdown() throws SQLException { if (connection != null) { connection.close(); } } // Get person list public List<Person> getPersonList() throws SQLException { try ( Statement stmnt = connection.createStatement(); ResultSet rs = stmnt.executeQuery("select * from person"); ) { List<Person> personList = new ArrayList<>(); while (rs.next()) { String firstName = rs.getString("first_name"); String lastName = rs.getString("last_name"); String email = rs.getString("email_address"); Person person = new Person(firstName, lastName, email); personList.add(person); } return personList; } } }
This class handles the connection establishment, query execution, and data retrieval. The getPersonList() method queries the "person" table in the database and converts the retrieved data into a list of Person objects.
Person Model (data representation):
import javafx.beans.property.StringProperty; import javafx.beans.property.SimpleStringProperty; public class Person { private StringProperty firstName = new SimpleStringProperty(this, "firstName"); public StringProperty firstNameProperty() { return firstName; } public String getFirstName() { return firstNameProperty().get(); } public void setFirstName(String firstName) { firstNameProperty().set(firstName); } private StringProperty lastName = new SimpleStringProperty(this, "lastName"); public StringProperty lastNameProperty() { return lastName; } public String getLastName() { return lastNameProperty().get(); } public void setLastName(String lastName) { lastNameProperty().set(lastName); } private StringProperty email = new SimpleStringProperty(this, "email"); public StringProperty emailProperty() { return email; } public String getEmail() { return emailProperty().get(); } public void setEmail(String email) { emailProperty().set(email); } public Person() {} public Person(String firstName, String lastName, String email) { setFirstName(firstName); setLastName(lastName); setEmail(email); } }
This class defines the Person object with properties for firstName, lastName, and email.
UI Class (displays data in a table):
import javafx.application.Application; import javafx.scene.control.TableView; import javafx.scene.control.TableColumn; import javafx.scene.control.cell.PropertyValueFactory; import javafx.scene.layout.BorderPane; import javafx.scene.Scene; import javafx.stage.Stage; public class PersonTableApp extends Application { private PersonDataAccessor dataAccessor; @Override public void start(Stage primaryStage) throws Exception { dataAccessor = new PersonDataAccessor(...); // Provide DB connection details TableView<Person> personTable = new TableView<>(); TableColumn<Person, String> firstNameCol = new TableColumn<>("First Name"); firstNameCol.setCellValueFactory(new PropertyValueFactory<>("firstName")); TableColumn<Person, String> lastNameCol = new TableColumn<>("Last Name"); lastNameCol.setCellValueFactory(new PropertyValueFactory<>("lastName")); TableColumn<Person, String> emailCol = new TableColumn<>("Email"); emailCol.setCellValueFactory(new PropertyValueFactory<>("email")); personTable.getColumns().addAll(firstNameCol, lastNameCol, emailCol); personTable.getItems().addAll(dataAccessor.getPersonList()); BorderPane root = new BorderPane(); root.setCenter(personTable); Scene scene = new Scene(root, 600, 400); primaryStage.setScene(scene); primaryStage.show(); } @Override public void stop() throws Exception { if (dataAccessor != null) { dataAccessor.shutdown(); } } public static void main(String[] args) { launch(args); } }
This class initializes the UI and displays the Person objects in a TableView.
By following these steps, you can create a JavaFX application that successfully connects to a MySQL database, retrieves data, and displays it in a user-friendly table.
The above is the detailed content of How to Connect a JavaFX Application to a MySQL Database and Display Data in a TableView?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










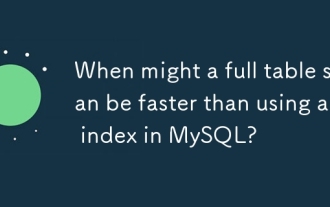
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
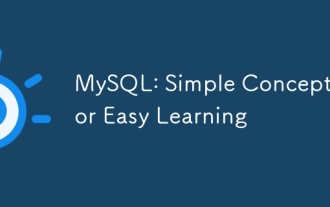
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
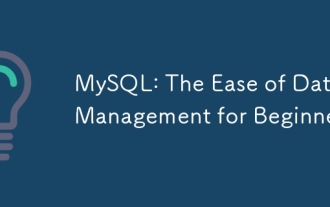
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
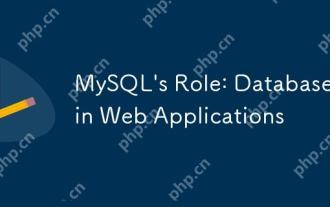
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
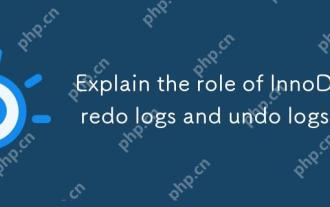
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
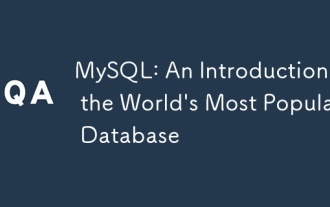
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
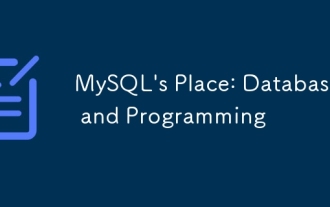
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
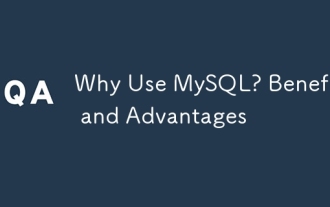
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
