


Why Does Python Throw an \'UnboundLocalError\' and How Can I Fix It?
Error: "UnboundLocalError: local variable referenced before assignment" in Python
When you encounter this error, it's because you're attempting to access a local variable before it's been initialized or defined. Let's explore the code that's causing this issue:
Var1 = 1 Var2 = 0 def function(): if Var2 == 0 and Var1 > 0: print("Result 1") elif Var2 == 1 and Var1 > 0: print("Result 2") elif Var1 < 1: print("Result 3") Var1 -= 1 function()
This code defines two variables, Var1 and Var2, outside of the function function(). Within the function, we're trying to manipulate Var1, which is fine. However, the problem arises when we access Var1 without initializing it within the function's scope.
Solution Using Global Variables:
To resolve this issue, we can declare Var1 as a global variable within the function. This tells Python to use the Var1 defined outside the function instead of creating a new local variable inside it. To achieve this, add the following line at the beginning of the function:
global Var1
Alternative Solution: Use Nonlocal Variables:
Python 3 introduces the nonlocal statement, which allows you to modify a variable defined in an enclosing scope. Instead of declaring Var1 as a global variable, we can use the following code within the function:
nonlocal Var1 Var1 -= 1
Conclusion:
UnboundLocalError occurs when you try to reference a local variable that hasn't been initialized or defined. To fix this, you can either declare the variable as global or use the nonlocal keyword to access the variable defined in an enclosing scope.
The above is the detailed content of Why Does Python Throw an \'UnboundLocalError\' and How Can I Fix It?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










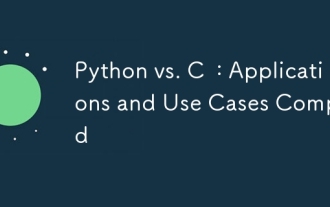
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
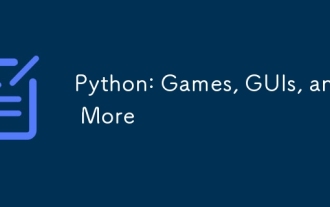
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
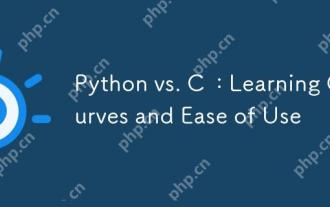
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
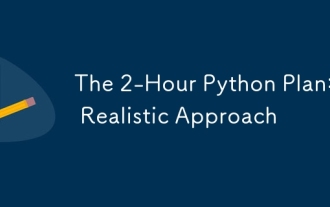
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
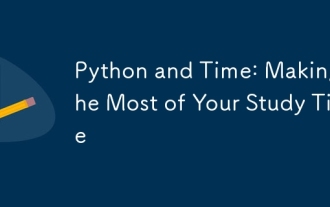
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
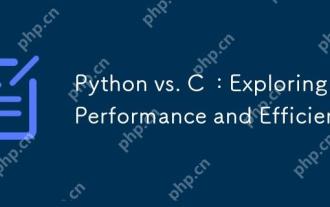
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
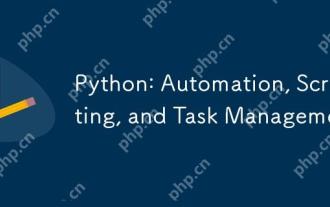
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
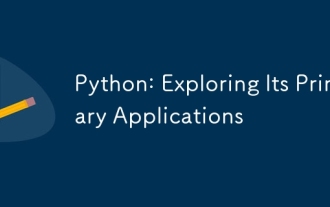
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
