


Mastering Asynchronous JavaScript: Callbacks, Promises, and Async/Await Simplified
Asynchronous JavaScript: From Callbacks to Promises and Async/Await
Introduction
JavaScript is a powerful, single-threaded programming language widely used for web development. A common challenge in JavaScript is handling asynchronous tasks, such as fetching data from an API or performing time-sensitive operations, without blocking the main thread. Over time, developers have moved from using callbacks to promises and now the more elegant async/await syntax to manage asynchronous operations. This guide will take you through these concepts step-by-step, starting from the basics and building up to advanced scenarios. By the end, you will be able to confidently use asynchronous JavaScript in real-world applications.
What Is Asynchronous Programming?
In JavaScript, tasks like fetching data from a server, reading files, or setting timeouts can take time to complete. Instead of waiting for these tasks to finish (which would block the execution of the rest of the program), JavaScript allows such tasks to run asynchronously. This means they are handled independently of the main program flow, allowing other code to execute without delay.
Understanding Callbacks: The Starting Point
What Are Callbacks?
A callback is a function passed as an argument to another function. When the first function completes its operation, it executes the callback function to signal completion.
Callback Example: Understanding Basics
function fetchData(callback) { setTimeout(() => { console.log("Data fetched!"); callback(); }, 2000); // Simulates a 2-second delay } function processData() { console.log("Processing data..."); } fetchData(processData);
Explanation:
- fetchData simulates fetching data with a delay.
- After the delay, it executes the callback function (processData), indicating that the data is ready.
Callback Hell: The Problem
Using nested callbacks to handle multiple asynchronous tasks can quickly lead to unreadable and hard-to-maintain code.
setTimeout(() => { console.log("Step 1: Data fetched"); setTimeout(() => { console.log("Step 2: Data processed"); setTimeout(() => { console.log("Step 3: Data saved"); }, 1000); }, 1000); }, 1000);
This "pyramid of doom" makes debugging and maintaining code difficult.
Promises: A Better Alternative
What Are Promises?
A promise is an object that represents a value that may be available now, in the future, or never. Promises have three states:
- Pending: Initial state, neither fulfilled nor rejected.
- Fulfilled: Operation completed successfully.
- Rejected: Operation failed.
Promise Example: Rewriting Callback Hell
function fetchData(callback) { setTimeout(() => { console.log("Data fetched!"); callback(); }, 2000); // Simulates a 2-second delay } function processData() { console.log("Processing data..."); } fetchData(processData);
Benefits of Promises
- Improved readability with chaining.
- Built-in error handling using .catch().
- Avoids deeply nested callbacks.
Async/Await: Modern Elegance
What Is Async/Await?
async/await is syntactic sugar over promises, introduced in ES2017. It makes asynchronous code look synchronous, improving readability and maintainability.
Async/Await Example: Simplifying Promises
setTimeout(() => { console.log("Step 1: Data fetched"); setTimeout(() => { console.log("Step 2: Data processed"); setTimeout(() => { console.log("Step 3: Data saved"); }, 1000); }, 1000); }, 1000);
How It Works
- async keyword: Declares a function as asynchronous.
- await keyword: Pauses the function execution until the promise resolves or rejects.
Benefits of Async/Await
- Sequential, readable code.
- Error handling with try...catch.
- Reduces callback complexity.
Handling Real-World Scenarios With Async/Await
Parallel Execution: Promise.all
When you need to execute multiple independent asynchronous tasks in parallel:
function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => { console.log("Data fetched!"); resolve("Fetched data"); }, 1000); }); } function processData(data) { return new Promise((resolve, reject) => { setTimeout(() => { console.log(`Processing: ${data}`); resolve("Processed data"); }, 1000); }); } function saveData(data) { return new Promise((resolve, reject) => { setTimeout(() => { console.log(`Saving: ${data}`); resolve("Data saved"); }, 1000); }); } // Chaining Promises fetchData() .then((data) => processData(data)) .then((processedData) => saveData(processedData)) .then((finalResult) => console.log(finalResult)) .catch((error) => console.error("Error:", error));
Error Handling: try...catch vs .catch()
Properly manage errors with try...catch for async functions:
async function handleData() { try { const fetchedData = await fetchData(); const processedData = await processData(fetchedData); const savedData = await saveData(processedData); console.log(savedData); } catch (error) { console.error("Error:", error); } } handleData();
Common FAQs About Asynchronous JavaScript
What Happens If I Forget await?
The function returns a promise instead of the resolved value. This may lead to unexpected behavior.
async function fetchAllData() { const task1 = fetchData(); const task2 = fetchData(); const results = await Promise.all([task1, task2]); console.log("All data fetched:", results); } fetchAllData();
Can I Use Async/Await Without Promises?
No, await works exclusively with promises. However, libraries like fetch and Node.js's fs.promises API provide native promise-based methods.
When Should I Use Callbacks?
Callbacks are still useful for small, simple tasks or when working with older APIs that don't support promises.
Conclusion
Mastering asynchronous JavaScript is essential for any developer working with modern web applications. Starting with callbacks, you can appreciate how promises and async/await simplify asynchronous code and improve readability. Use callbacks sparingly, leverage promises for better error handling, and embrace async/await for clean, intuitive code. Armed with these tools, you'll be ready to tackle any asynchronous challenge in your projects.
The above is the detailed content of Mastering Asynchronous JavaScript: Callbacks, Promises, and Async/Await Simplified. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










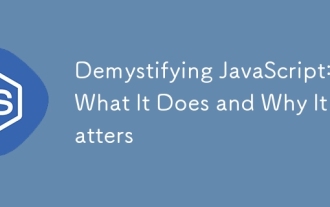
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
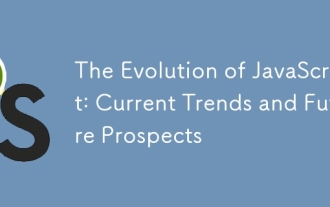
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
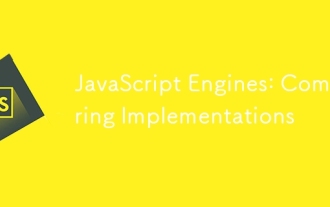
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
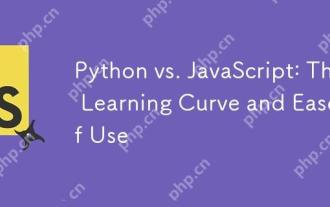
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
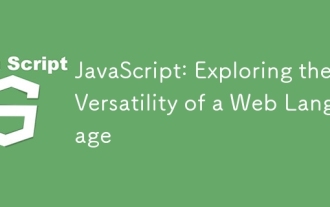
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
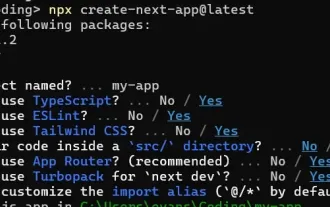
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
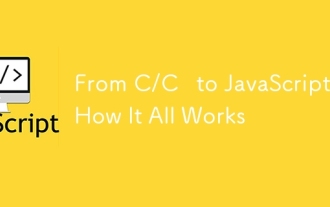
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
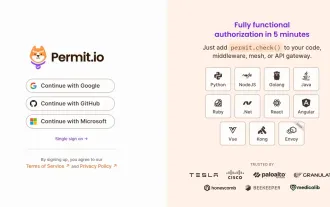
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
