


How Can I Reliably Determine if a Facebook User Has Liked My Page Using JavaScript?
Determining Facebook Page Affinity with JavaScript API
In the context of iFrame applications, the task of verifying whether a user has "liked" a particular Facebook page can be encountered. However, encountering inconsistencies while attempting to implement this functionality is not uncommon.
Consider the following code snippet:
FB.api({ method: "pages.isFan", page_id: my_page_id, }, function(response) { console.log(response); if(response){ alert('You Likey'); } else { alert('You not Likey :('); } } );
Upon executing this code, it yields a puzzling result of "False" even though the user in question has indeed "liked" the associated page. The culprit behind this discrepancy lies in the need for an extended permission that has not been addressed.
Alternative Approach Using Signed Request
To circumvent this issue and reliably ascertain a user's "like" status, an alternative approach utilizing the signed request mechanism can be employed. By enabling the "OAuth 2.0 for Canvas" advanced option within Facebook, you can retrieve a signed request with every tab app request that contains crucial information about the user, including their "like" status:
function parsePageSignedRequest() { if (isset($_REQUEST['signed_request'])) { $encoded_sig = null; $payload = null; list($encoded_sig, $payload) = explode('.', $_REQUEST['signed_request'], 2); $sig = base64_decode(strtr($encoded_sig, '-_', '+/')); $data = json_decode(base64_decode(strtr($payload, '-_', '+/'), true)); return $data; } return false; } if($signed_request = parsePageSignedRequest()) { if($signed_request->page->liked) { echo "This content is for Fans only!"; } else { echo "Please click on the Like button to view this tab!"; } }
This solution extracts the signed request, decodes it, and examines the "liked" property of the parsed JSON object to accurately determine the user's "like" status for your Facebook page.
The above is the detailed content of How Can I Reliably Determine if a Facebook User Has Liked My Page Using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
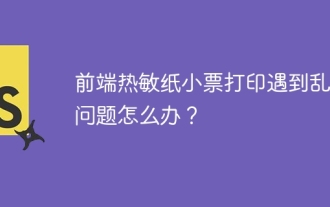
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
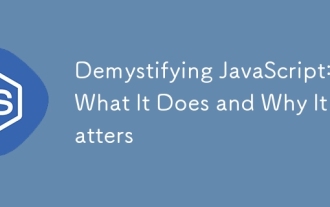
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
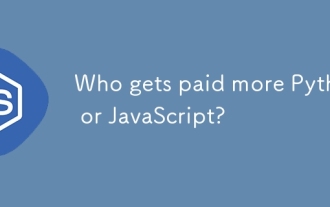
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
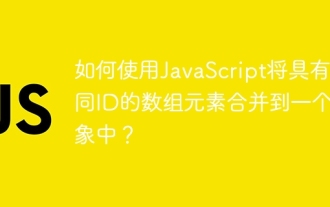
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
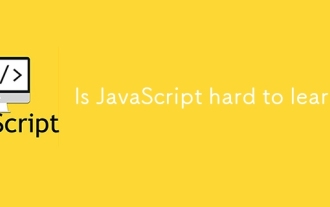
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
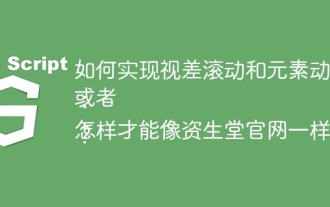
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
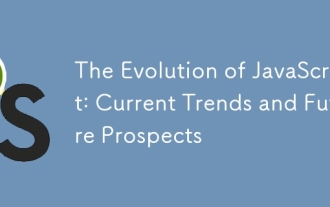
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
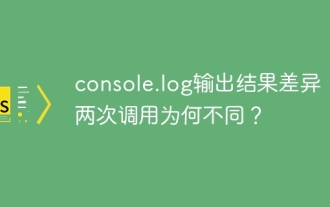
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
