Why Are My Go Variables Undefined, and How Can I Fix This?
Understanding Undefined Variables in Go
When attempting to compile your Go program, you encountered the errors "undefined err" and "undefinded user." This can be perplexing, especially for a beginner navigating the nuances of the language.
The issue arises from the limited scope of variables declared within blocks. In your original code, you declare "err" and "user" inside the if-else block. However, these variables are only visible within that specific block. When you attempt to access them outside the block, the compiler complains because they are undefined.
Here's how the scope of variables works in Go:
- Variables declared outside a block are visible throughout the function.
- Variables declared within a block (like the if-else block) are only visible within that block.
To resolve this issue, move the declarations of "err" and "user" outside the if-else block, ensuring they have validity throughout the function. You can do this by declaring them before the if statement, for example:
var err error var user core.User if req.Id == nil { user, err = signup(C, c, &req) } else { user, err = update(C, c, &req) }
This ensures that "err" and "user" are defined before their use and are available in subsequent code blocks.
In your updated code, you introduce another variable declaration issue. Using the short variable declaration user := core.User{} within the if block creates new variables that are not visible outside that block. As a result, the compiler complains that the "user" declared outside the block is not used.
To resolve this, move the declaration of "user" before the if block and use the assignment operator:
user := core.User{} if req.Id == nil { user, err = signup(C, c, &req) } else { user, err = update(C, c, &req) }
Alternatively, you can use a single line to declare both "user" and "err" and initialize them with default values:
user, err := core.User{}, error(nil)
By understanding the scope of variables in Go, you can avoid these common compilation errors and write more efficient and maintainable code.
The above is the detailed content of Why Are My Go Variables Undefined, and How Can I Fix This?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










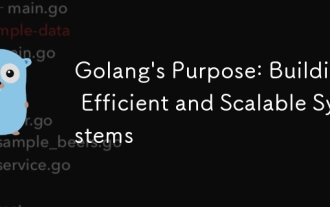
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
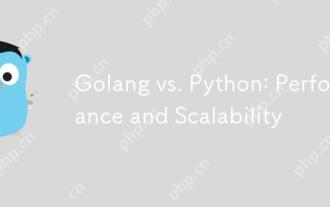
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
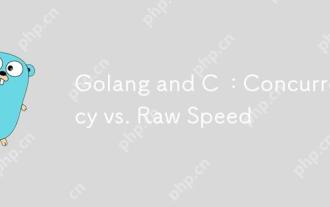
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
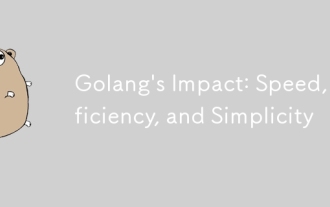
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
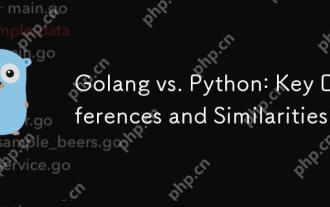
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
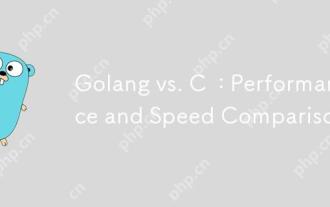
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
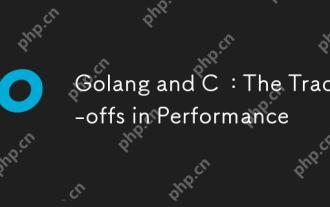
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
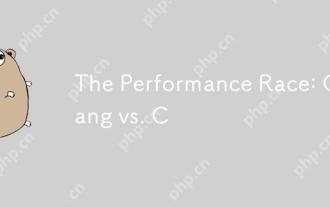
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
