


How Can I Efficiently Execute Parameterized SELECT Queries and Use Their Results for INSERT Operations with PDO?
Efficient Parameterized SELECT Queries with PDO
In database programming, parameterized queries are essential for ensuring data security and performance. PDO (PHP Data Objects) provides a robust framework for executing parameterized queries.
Executing a Parameterized SELECT Query
To execute a parameterized SELECT query, follow these steps:
- Prepare the statement: Use the prepare() method to create a PDOStatement object. This statement includes placeholders (named parameters) for input values.
$db = new PDO("..."); $statement = $db->prepare("SELECT id FROM some_table WHERE name = :name");
- Bind parameters: Call the bindValue() or bindParam() method to bind specific values to placeholders.
$name = "Jimbo"; $statement->bindParam(':name', $name);
- Execute the statement: Invoke the execute() method to execute the query.
$statement->execute();
- Fetch results: Use the fetch() or fetchAll() method to retrieve the query results.
$row = $statement->fetch();
Inserting Data Based on SELECT Query Results
In your case, you want to insert data into another table based on the ID obtained from the SELECT query.
- Prepare the INSERT statement: Create a separate PDOStatement for the INSERT query.
$insertStatement = $db->prepare("INSERT INTO some_other_table (some_id) VALUES (:some_id)");
- Bind the ID parameter: Obtain the ID from the SELECT query results and bind it to the INSERT statement.
$someId = $row['id']; $insertStatement->bindParam(':some_id', $someId);
- Execute the INSERT query: Call the execute() method to insert the data.
$insertStatement->execute();
Exception Handling
To simplify error handling, consider enabling PDO exceptions:
$db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
This configuration will throw a PDOException if any query fails, eliminating the need for explicit error checks.
Preparing Queries for Reuse
Prepared statements can be beneficial when executing the same query repeatedly, as they reduce compilation time. However, because PDO provides efficient query execution, the advantage of prepared queries is generally marginal.
The above is the detailed content of How Can I Efficiently Execute Parameterized SELECT Queries and Use Their Results for INSERT Operations with PDO?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










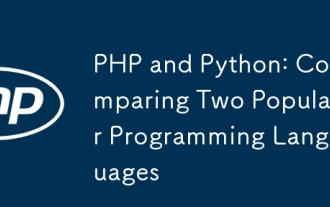
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
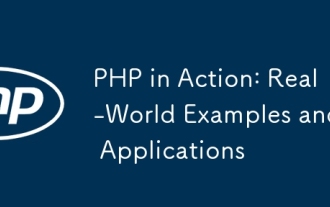
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
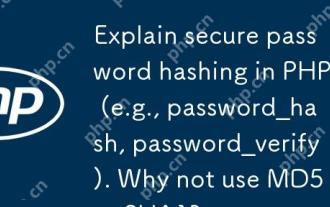
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
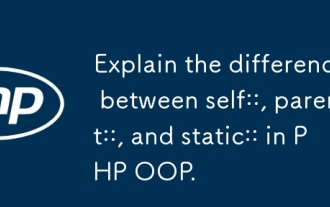
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
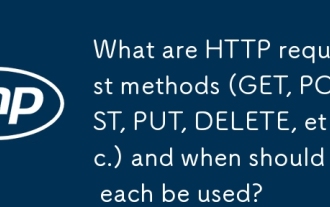
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
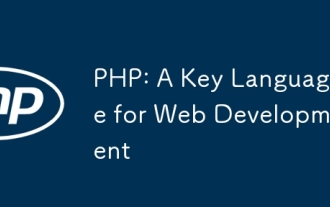
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
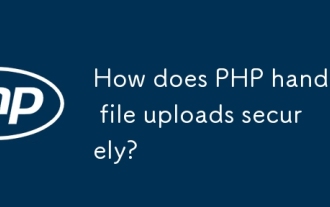
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
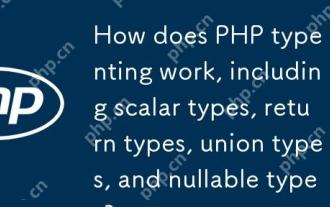
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
