How to Convert PySpark String Columns to Date Format?
Converting PySpark String to Date Format
You have a PySpark DataFrame with a string column representing dates in the MM-dd-yyyy format. Your attempt to convert this column to a date format using to_date function returns nulls. This article provides methods for addressing this issue.
Updated Recommendation (Spark 2.2 ):
For Spark versions 2.2 and above, the preferred approach is to use the to_date or to_timestamp functions, which now support the format argument. This allows you to specify the input format and convert the string column directly to a date or timestamp:
from pyspark.sql.functions import to_timestamp df = spark.createDataFrame([('1997-02-28 10:30:00',)], ['t']) df.select(to_timestamp(df.t, 'yyyy-MM-dd HH:mm:ss').alias('dt')).collect() # Output: # [Row(dt=datetime.datetime(1997, 2, 28, 10, 30))]
Original Answer (Spark < 2.2):
For earlier Spark versions, you can use the following method without the need for a user-defined function (UDF):
from pyspark.sql.functions import unix_timestamp, from_unixtime df = spark.createDataFrame( [("11/25/1991",), ("11/24/1991",), ("11/30/1991",)], ['date_str'] ) df2 = df.select( 'date_str', from_unixtime(unix_timestamp('date_str', 'MM/dd/yyy')).alias('date') ) print(df2) # Output: # DataFrame[date_str: string, date: timestamp] df2.show(truncate=False) # Output: # +----------+-------------------+ # |date_str |date | # +----------+-------------------+ # |11/25/1991|1991-11-25 00:00:00| # |11/24/1991|1991-11-24 00:00:00| # |11/30/1991|1991-11-30 00:00:00| # +----------+-------------------+
In this method, unix_timestamp converts the string column to a Unix timestamp, and from_unixtime converts the Unix timestamp to a date column.
The above is the detailed content of How to Convert PySpark String Columns to Date Format?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


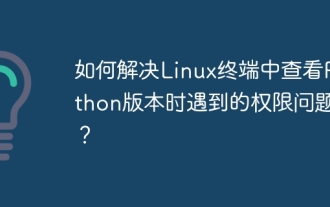
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
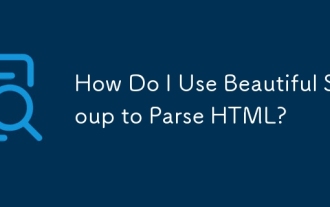
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
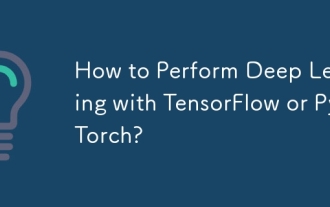
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
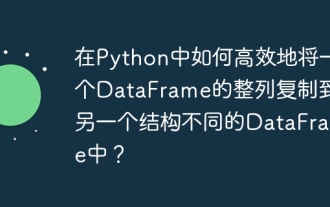
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
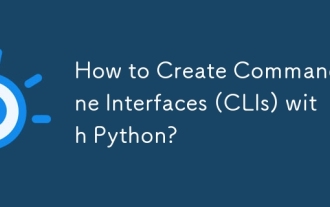
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
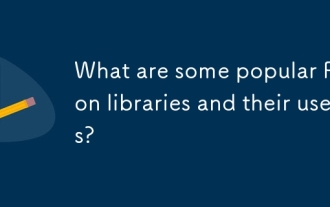
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
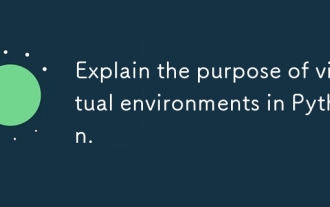
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.
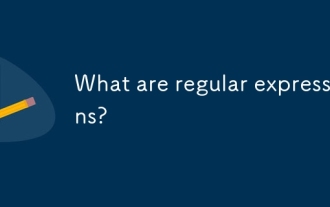
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
