Why Did Google Directions API Stop Providing KML Data?
Why Google Directions API no longer provides KML data?
Google Directions API stopped providing KML data since July 27th, 2012. Therefore, developers need to migrate to JSON or XML formats instead.
Alternatives:
JSON:
To parse JSON data, you can create six classes as follows:
Parser.java: Defines an interface for parsing.
XMLParser.java: Provides a base class for XML parsing.
Segment.java: Represents a segment of the route.
Route.java: Represents the entire route.
GoogleParser.java: Parses Google JSON data.
RouteOverlay.java: Draws the route on a map overlay.
XML:
Alternatively, you can use XML for parsing, replacing GoogleParser.java with the following:
XMLParser.java: Parses Google XML data.
Code Implementation:
To use these classes, you can create the following code:
private Route directions(GeoPoint start, GeoPoint dest) { Parser parser; String jsonURL = "https://developers.google.com/maps/documentation/directions/#JSON"; // API URL StringBuffer sBuf = new StringBuffer(jsonURL); sBuf.append("?origin="); sBuf.append(start.getLatitudeE6()/1E6); sBuf.append(','); sBuf.append(start.getLongitudeE6()/1E6); sBuf.append("&destination="); sBuf.append(dest.getLatitudeE6()/1E6); sBuf.append(','); sBuf.append(dest.getLongitudeE6()/1E6); sBuf.append("&sensor=true&mode=driving"); parser = new GoogleParser(sBuf.toString()); Route r = parser.parse(); return r; }
Then, add the following code to your onCreate() function:
MapView mapView = (MapView) findViewById(R.id.mapview); Route route = directions(new GeoPoint((int)(26.2*1E6),(int)(50.6*1E6)), new GeoPoint((int)(26.3*1E6),(int)(50.7*1E6))); RouteOverlay routeOverlay = new RouteOverlay(route, Color.BLUE); mapView.getOverlays().add(routeOverlay); mapView.invalidate();
The above is the detailed content of Why Did Google Directions API Stop Providing KML Data?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










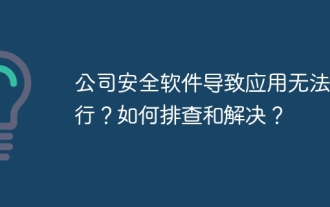
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
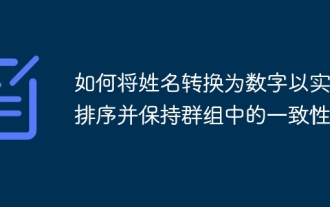
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
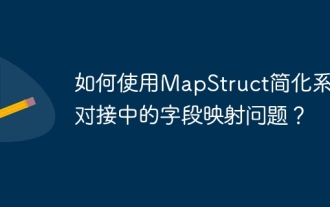
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
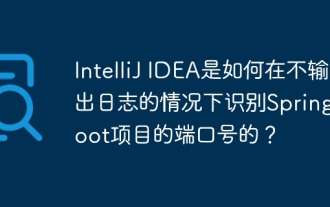
Start Spring using IntelliJIDEAUltimate version...
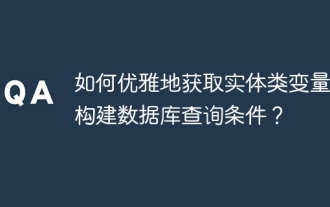
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
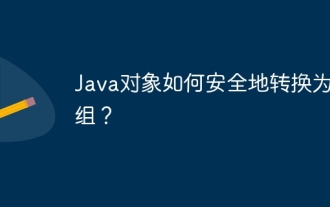
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
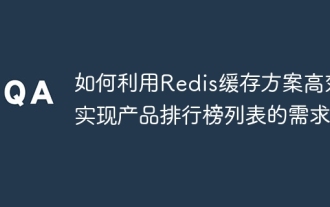
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
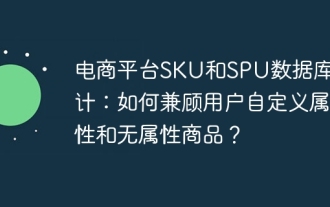
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
