


How Does Matplotlib\'s `pyplot.scatter()` Function Use the `s` Parameter to Control Marker Size?
pyplot Scatter Plot Marker Size
In the matplotlib.pyplot.scatter() function, the s parameter specifies the marker size. This size is defined in "points^2," which can be a confusing unit of measurement to interpret.
What is a "Point"?
A "point" in this context is an arbitrary unit of measurement used for defining the size of markers. It is not directly related to the size of a pixel on your display.
How Does s Affect Marker Size?
The s parameter specifies the area of the marker. This means that:
- Increasing s by a factor of 4 increases the width and height of the marker by a factor of 2.
- Doubling the width of the marker (or any linear dimension) requires increasing s by a factor of 4.
- Doubling the area of the marker requires increasing s by a factor of 2.
Example
Let's create a scatter plot with different marker sizes:
import matplotlib.pyplot as plt x = [0, 2, 4, 6, 8, 10] y = [0] * len(x) s = [20 * 4**n for n in range(len(x))] plt.scatter(x, y, s=s) plt.show()
In this example, the marker size increases exponentially as we move from left to right. Each marker has twice the area of the previous marker.
Visualizing Marker Size
To visualize the different functions that affect marker size, let's create the following plot:
import matplotlib.pyplot as plt x = [0, 2, 4, 6, 8, 10, 12, 14, 16, 18] s_exp = [20 * 2**n for n in range(len(x))] s_square = [20 * n**2 for n in range(len(x))] s_linear = [20 * n for n in range(len(x))] plt.scatter(x, [1] * len(x), s=s_exp, label='$s=2^n$', lw=1) plt.scatter(x, [0] * len(x), s=s_square, label='$s=n^2$') plt.scatter(x, [-1] * len(x), s=s_linear, label='$s=n$') plt.ylim(-1.5, 1.5) plt.legend(loc='center left', bbox_to_anchor=(1.1, 0.5), labelspacing=3) plt.show()
This plot demonstrates how the marker size appears when scaled exponentially, quadratically, and linearly.
The above is the detailed content of How Does Matplotlib\'s `pyplot.scatter()` Function Use the `s` Parameter to Control Marker Size?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










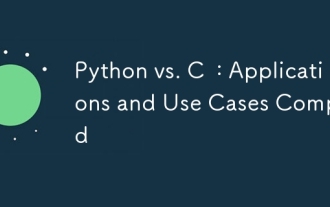
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
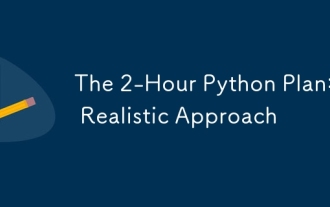
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
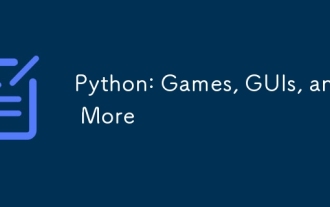
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
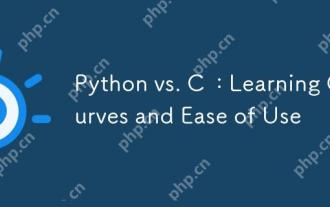
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
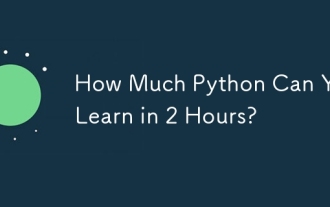
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
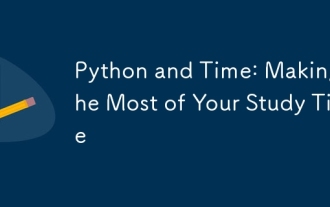
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
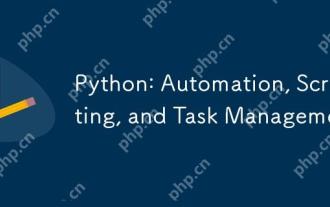
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
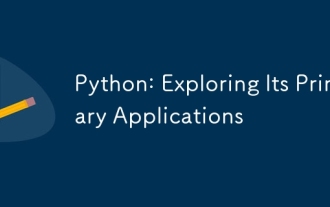
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
