How to Modify Specific Lines in Text Files with Python?
Editing Specific Lines in Text Files with Python
In this code snippet, you're trying to modify a specific line in 'stats.txt', but the approach you're using is incorrect. Fortunately, there's a Pythonic way to accomplish this task.
Firstly, open the file using a 'with' statement:
with open('stats.txt', 'r') as file:
This ensures that the file is properly handled and closed when the operation is complete.
Next, read all the lines of the file into a list using 'readlines()':
data = file.readlines()
This effectively loads the entire file contents into memory.
Now, you can modify the desired line by indexing the 'data' list:
data[1] = 'Mage\n' # Change "Warrior" in line 2 to "Mage"
Finally, write the modified list of lines back to the file:
with open('stats.txt', 'w') as file: file.writelines(data)
By loading the entire file into memory, you can manipulate specific lines effectively without having to overwrite the entire file. This technique is particularly useful for making multiple modifications to a file or if you need to preserve the file's existing contents.
The above is the detailed content of How to Modify Specific Lines in Text Files with Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
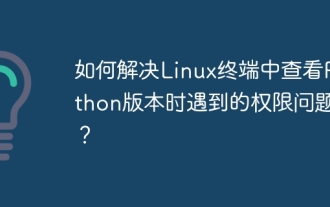
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
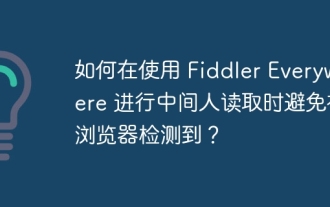
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
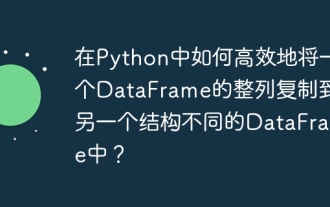
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
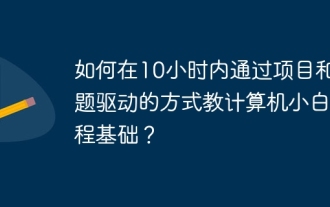
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
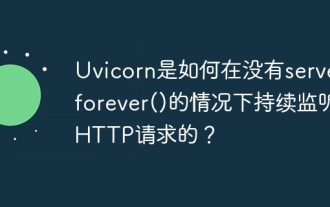
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
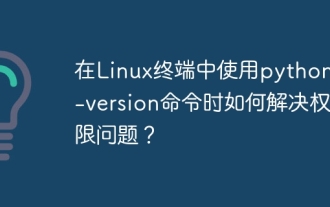
Using python in Linux terminal...
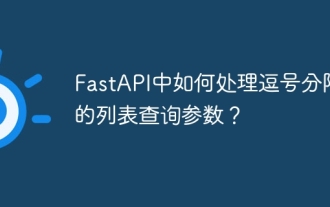
Fastapi ...
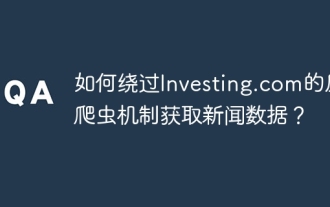
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
