How Can I Retrieve a Random Value from an Array in PHP?
Nov 25, 2024 pm 04:43 PMRandom Array Value Retrieval
When working with arrays, selecting random values can enhance your coding. Let's explore how to achieve this using PHP's built-in functions.
Getting a Random Array Value
You have an array named $ran = array(1,2,3,4). To obtain a random value from this array and store it in a variable, follow these steps:
- Use the array_rand() function to generate a random index for the array.
- Specify the array name, $ran, as the argument within the parentheses.
- Assign the result to a variable, such as $index.
$index = array_rand($ran);
The generated index represents the position of the randomly selected value in the $ran array.
To obtain the actual random value, access the element in the array at the generated index. You can achieve this using array indexing:
$random_value = $ran[$index];
Now, the $random_value variable contains the randomly selected value from the $ran array.
Variant Method for Associative Arrays
If you have an associative array, an alternative method exists while utilizing array_rand(). You can retrieve both the index and value through the following steps:
- Generate a random index using array_rand() as described earlier.
- Assign the result to a variable, such as $index.
- Retrieve the value from the associative array using the $index as the key.
$index = array_rand($array); $v = $array[$index];
This provides you with the ability to obtain the value attached to the randomly selected index in an associative array.
By leveraging these techniques, you can effectively generate random values from arrays in PHP, enhancing the versatility of your code.
The above is the detailed content of How Can I Retrieve a Random Value from an Array in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
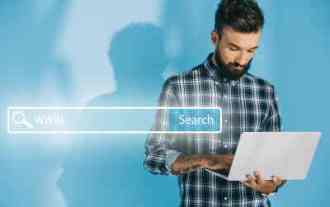
11 Best PHP URL Shortener Scripts (Free and Premium)
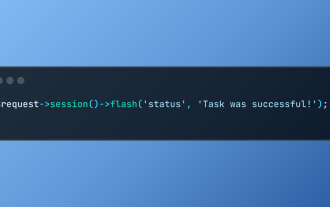
Working with Flash Session Data in Laravel
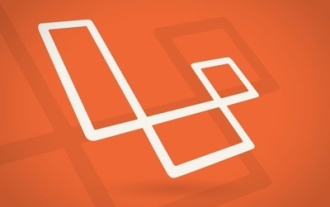
Build a React App With a Laravel Back End: Part 2, React
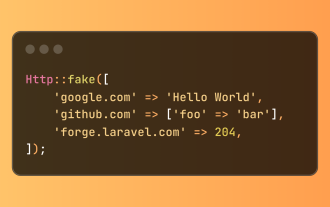
Simplified HTTP Response Mocking in Laravel Tests
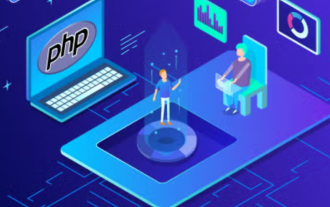
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
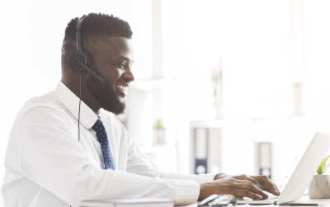
12 Best PHP Chat Scripts on CodeCanyon
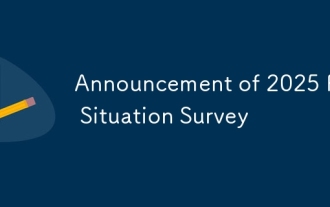
Announcement of 2025 PHP Situation Survey
