


How to Efficiently Add Ordinal Suffixes (st, nd, rd, th) to Numbers in PHP?
Displaying Numbers with Ordinal Suffixes in PHP
When displaying numbers in certain contexts, it is necessary to append the appropriate ordinal suffix (st, nd, rd, or th) to indicate their order. This can be challenging, especially for large or complex numbers.
Finding the Correct Suffix
To determine the correct ordinal suffix for a number, one can utilize an array-based approach. This involves storing the suffixes in an array and selecting the appropriate one based on the last digit of the number.
Code Implementation
The following PHP code snippet demonstrates how to implement the ordinal suffix array method:
$ends = array('th', 'st', 'nd', 'rd', 'th', 'th', 'th', 'th', 'th', 'th'); if (($number % 100) >= 11 && ($number % 100) <= 13) { $abbreviation = $number . 'th'; } else { $abbreviation = $number . $ends[$number % 10]; }
In this code, the $ends array contains the ordinal suffixes from 0th to 9th. The logic checks whether the number's last two digits are between 11 and 13, in which case it assigns 'th' as the suffix. Otherwise, it selects the suffix based on the last digit using the modulo operator (%).
Function-Based Approach
Alternatively, you can create a function to simplify the ordinal suffix calculation:
function ordinal($number) { $ends = array('th', 'st', 'nd', 'rd', 'th', 'th', 'th', 'th', 'th', 'th'); if ((($number % 100) >= 11) && (($number % 100) <= 13)) { return $number . 'th'; } else { return $number . $ends[$number % 10]; } } // Example usage: echo ordinal(100);
Conclusion
By utilizing the array-based or function-based approach, you can effortlessly display numbers with the correct ordinal suffixes, ensuring clarity and accuracy in your code.
The above is the detailed content of How to Efficiently Add Ordinal Suffixes (st, nd, rd, th) to Numbers in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


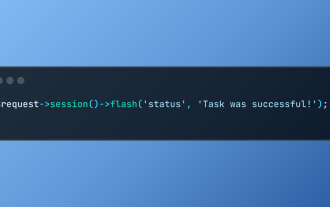
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
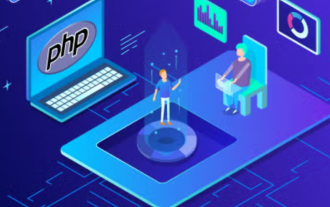
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
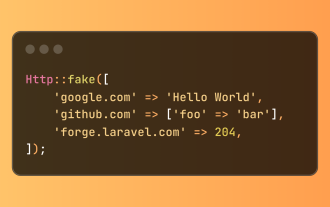
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
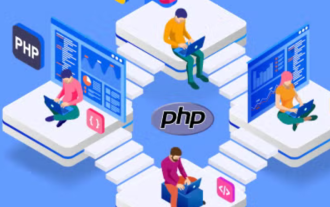
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
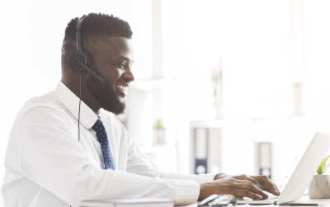
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
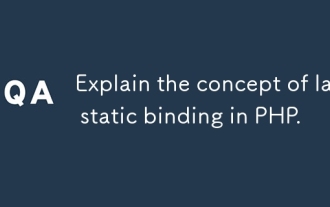
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
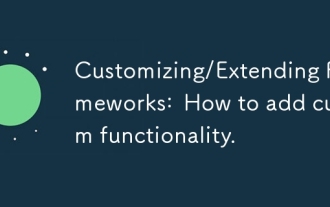
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
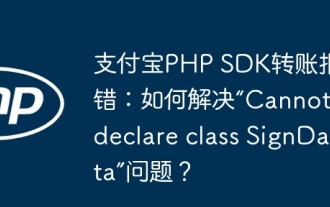
Alipay PHP...
