Iterators vs. Generators in Python: When to Use Which?
Generators vs. Iterators in Python
Python's iterators and generators are both iterables, but they differ in their implementation and use cases.
Iterators
Iterators are iterable objects that provide an interface for traversing a sequence of items. They have two main methods:
- __iter__: Returns the iterator itself, allowing it to be iterated over multiple times.
- __next__: Returns the next item in the sequence. Raising StopIteration when there are no more items left.
Generators
Generators are a special type of iterator that use the yield keyword to generate values on the fly. When called, a generator function returns a generator object that can be iterated over.
Internally, a generator stores a suspended execution state that keeps track of the current position in the iteration. When iterating over a generator, the __next__ method resumes the suspended function and yields the next value. The execution is then suspended again until the next iteration.
Use Cases
-
Use Iterators:
- When you need to maintain state across iterations (e.g., a custom iterator with complex behavior).
- When you need to expose additional methods besides iteration (e.g., a class with current() and next() methods).
-
Use Generators:
- When simplicity and efficiency are priorities.
- When you want to generate values lazily, without storing an intermediate list.
- When you want to pause and resume the iteration (e.g., suspending a computation for later use).
Example
Consider the following function that generates square numbers for a given range:
def squares(start, stop): for i in range(start, stop): yield i * i
This function creates a generator that yields square numbers one at a time. It's more efficient than a list comprehension or a custom iterator, as it avoids creating an intermediate list of all the squared values.
The above is the detailed content of Iterators vs. Generators in Python: When to Use Which?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










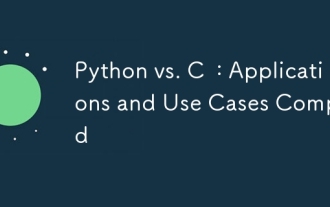
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
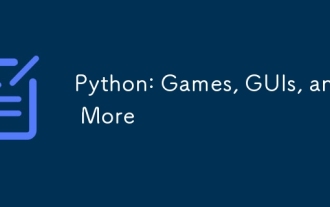
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
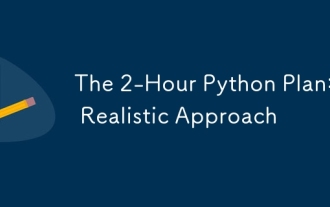
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
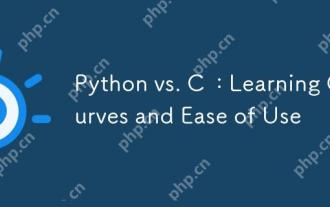
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
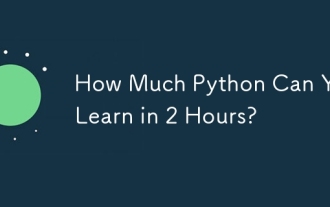
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
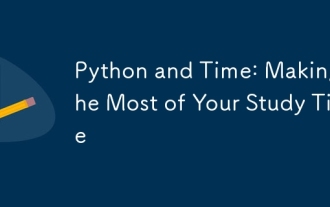
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
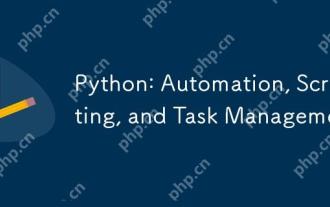
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
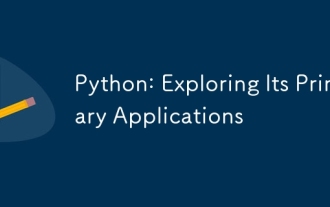
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
