


How Can I Get All HTML Element Attributes Using JavaScript or jQuery?
Getting All HTML Element Attributes using JavaScript/jQuery
As a developer, you may encounter situations where you need to extract all attributes associated with a specific HTML element into an array. This can be accomplished using both JavaScript and jQuery.
JavaScript Approach
To retrieve attribute names using pure JavaScript, you can leverage the attributes node list on the target element:
const element = document.getElementById("someId"); // Create an empty array to store attribute names const attributes = []; // Iterate over the attributes node list for (let i = 0; i < element.attributes.length; i++) { // Extract the attribute name attributes.push(element.attributes[i].nodeName); }
This approach provides an array containing only the attribute names.
jQuery Approach
jQuery offers simpler methods to extract HTML element attributes. Its attr() function returns all attributes as key-value pairs:
const element = $("#someId"); // Get all attribute names and values const attributes = {}; $.each(element.attr(), function (key, value) { attributes[key] = value; });
This code assigns all attribute names and values to a JavaScript object.
Considerations
When using the JavaScript approach, keep in mind that it only extracts attribute names. For attribute values, you would need to access the nodeValue property of each attribute node. The jQuery method, on the other hand, provides both attribute names and values.
Additionally, the number of attributes and their names can vary dynamically. This code handles the generic case of extracting all attributes from an element without relying on specific attribute names or count.
The above is the detailed content of How Can I Get All HTML Element Attributes Using JavaScript or jQuery?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
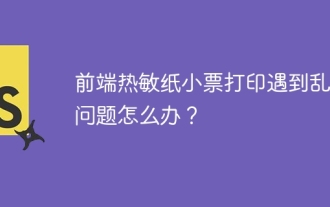
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
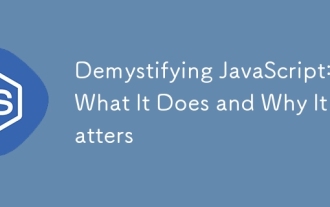
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
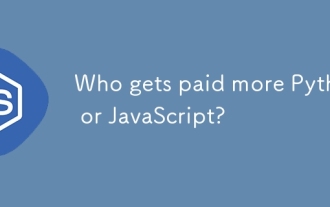
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
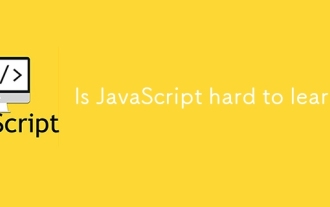
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
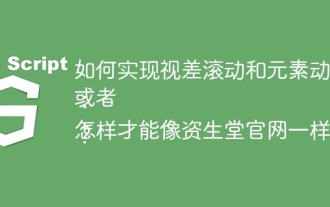
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
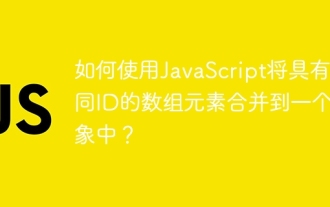
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
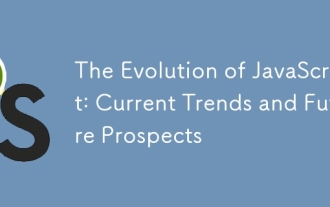
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
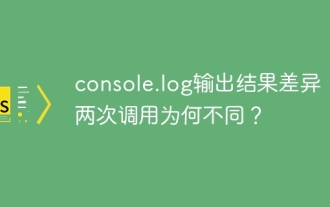
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
