How to Dial a Network Connection Using a Specific Interface in Go?
Dialing with a Specific Network Interface in Go
In Go, when establishing a network connection using the Dial function, it's possible to specify a specific network interface to use for outgoing traffic. This is particularly useful when multiple network interfaces are present on a system and you want to control which one is utilized for a particular connection.
To dial a connection using a specific network interface, you need to first retrieve the interface object using the InterfaceByName function. This function takes the name of the interface as its argument and returns an Interface object representing that interface.
Once you have the Interface object, you can access its list of addresses using the Addrs method. This method returns a slice of net.Addr objects, each of which represents an address assigned to that interface.
To use a specific address for dialing, you can extract it from the list of addresses returned by the Addrs method. However, it's important to note that the addresses returned by Addrs are of type *net.IPNet, which includes both an IP address and a netmask. For dialing purposes, you need to create a new net.TCPAddr object using the IP address portion of the *net.IPNet object and port number of the destination.
Here's an example code that demonstrates how to dial a connection using a specific network interface:
package main import ( "net" "log" ) func main() { // Get the interface by name. ief, err := net.InterfaceByName("eth1") if err != nil { log.Fatal(err) } // Get the list of addresses assigned to the interface. addrs, err := ief.Addrs() if err != nil { log.Fatal(err) } // Extract the IP address from the first address. ip := addrs[0].(*net.IPNet).IP // Create a new TCP address using the extracted IP address and destination port. tcpAddr := &net.TCPAddr{ IP: ip, Port: 80, } // Create a dialer with the specified local address. d := net.Dialer{LocalAddr: tcpAddr} // Dial the connection using the specified dialer. conn, err := d.Dial("tcp", "google.com:80") if err != nil { log.Fatal(err) } // Use the connection as needed. _ = conn }
By following these steps, you can establish a network connection using a specific network interface, allowing you to control the path of outgoing traffic.
The above is the detailed content of How to Dial a Network Connection Using a Specific Interface in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










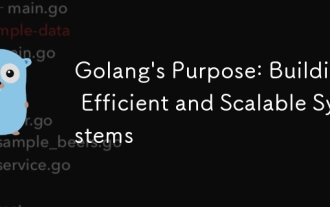
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
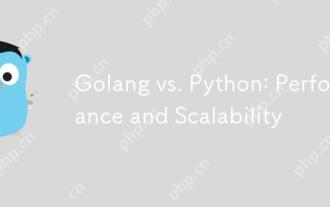
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
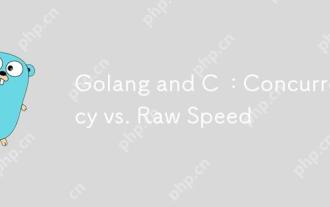
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
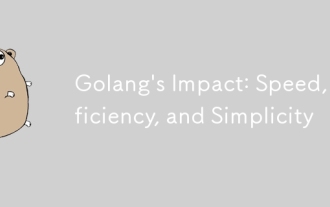
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
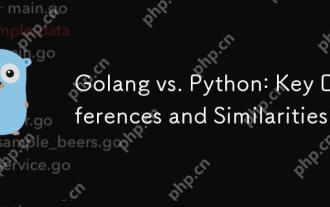
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
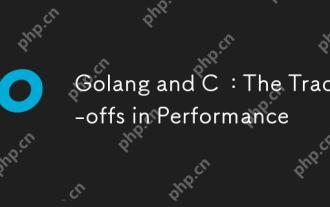
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
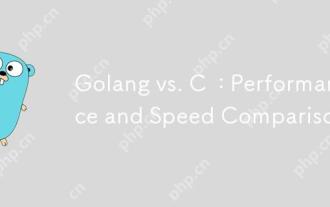
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
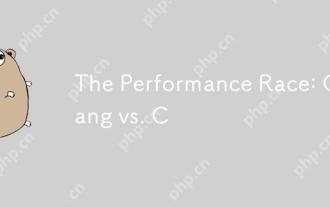
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
