How to Create Foreign Key Relationships in Golang using GORM?
Database Relationships with GORM: Creating Foreign Keys
In the realm of relational databases, foreign keys serve as crucial links between related tables. This article aims to guide you on how to establish a foreign key relationship in your Golang application using GORM, a popular ORM (Object-Relational Mapping) library.
Understanding the Problem
You have defined two models, User and UserInfo, and wish to establish a foreign key relationship between their UID and ID fields. The challenge lies in ensuring that the UID field in the UserInfo model correctly references the id field in the User model.
Solution
To resolve this issue, you need to configure a foreign key constraint in your database schema after defining your models. This can be achieved using the following steps:
1. Add Foreign Key During Migration
After creating the models, use GORM's AddForeignKey method to specify the foreign key relationship in your database migration. Add the following line to your migration code:
db.Model(&models.UserInfo{}).AddForeignKey("u_id", "t_user(id)", "RESTRICT", "RESTRICT")
This line establishes a foreign key constraint on the UID column in the t_user_info table, referencing the id column in the t_user table. It also sets the behavior of the foreign key to "RESTRICT", which will prevent the insertion or deletion of data that would violate the relationship.
2. Set Association Foreign Key
In your User model, you have annotated the User field in the UserInfo model with a foreign key tag:
User User `gorm:"foreignkey:u_id;association_foreignkey:id"`
This tag informs GORM that the User field should be used as the foreign key, and that it is associated with the id field in the User model.
3. Update Database
Run the database migration to apply the foreign key constraint to your schema.
Note:
Starting with GORM version 2.0, it is no longer necessary to explicitly set foreign key constraints in the model annotations. However, for earlier versions, the solution outlined above still applies.
By following these steps, you have established a proper foreign key relationship between your User and UserInfo models, ensuring that the UID field in the UserInfo model accurately references the data in the User table.
The above is the detailed content of How to Create Foreign Key Relationships in Golang using GORM?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










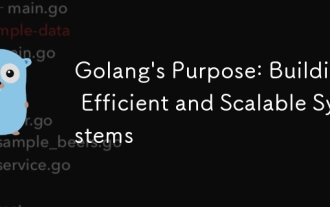
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
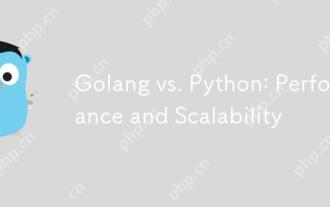
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
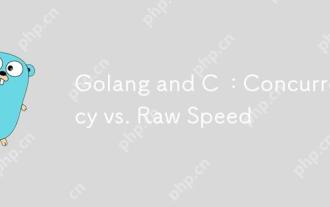
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
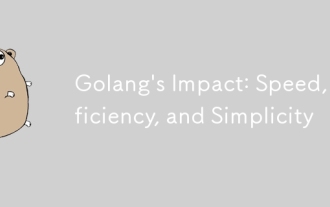
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
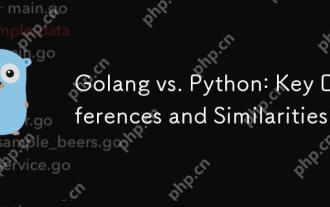
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
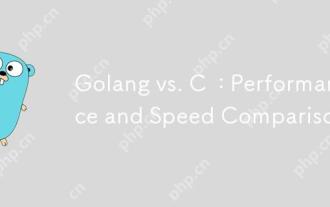
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
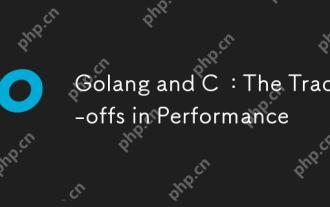
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
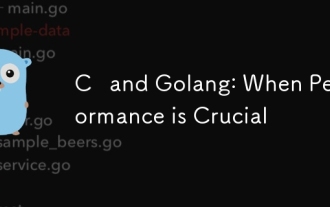
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
