


How Can I Create a Slice of Functions with Different Signatures in Go?
Creating a Slice of Functions with Different Signatures
Problem
How can you create a slice of functions with different signatures in Go?
Context
Go's type system is statically typed, which means that functions must have a fixed signature at compile time. However, it can be useful to create slices of functions that can accept arguments of different types or numbers.
Solution
While the provided code is functional, it requires using a switch statement to handle each function signature type. A more concise and flexible solution is to use reflection.
Here's an example:
package main import ( "fmt" "reflect" ) type Executor func(...interface{}) func main() { functions := []Executor{ func(a, b int) { fmt.Println(a + b) }, func(s string) { fmt.Println(s) }, func() { fmt.Println("No arguments") }, } for _, f := range functions { numIn := reflect.TypeOf(f).NumIn() args := make([]reflect.Value, numIn) for i := 0; i < numIn; i++ { switch reflect.TypeOf(f).In(i).Kind() { case reflect.Int: args[i] = reflect.ValueOf(12) case reflect.String: args[i] = reflect.ValueOf("Hello") default: args[i] = reflect.Value{} } } f.Call(args) } }
In this solution, we create a slice of Executor functions, which are functions that accept any number of arguments. The reflect package is used to determine the number and types of arguments expected by each function and generate the corresponding reflect.Value slice.
Using reflection allows us to dynamically call functions with varying signatures without the need for a type switch or interface{} slices.
The above is the detailed content of How Can I Create a Slice of Functions with Different Signatures in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










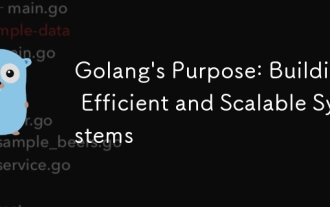
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
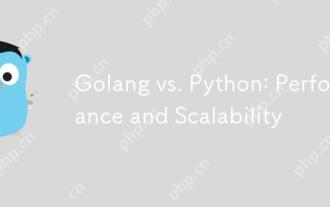
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
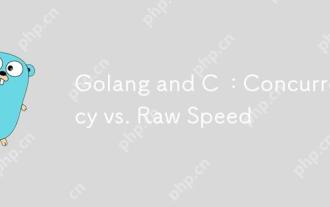
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
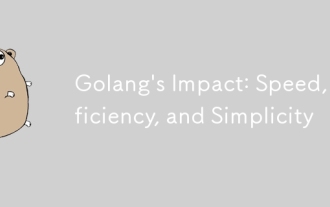
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
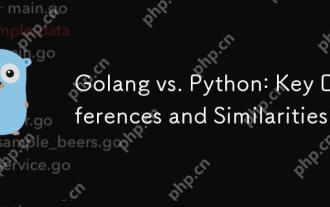
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
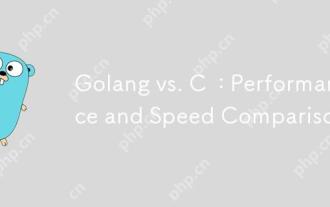
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
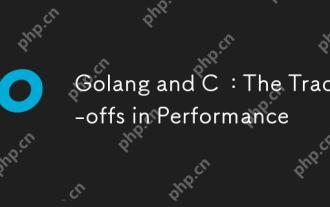
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
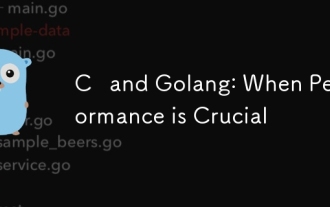
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
