


How Can a Custom Iterator Simplify Iterating Through Nested Containers?
Nov 26, 2024 am 05:36 AMFlattening Nested Containers with a Custom Iterator
Introduction
Iterating through nested containers can often be a tedious and error-prone task. To simplify this process, we explore the concept of a "flattening" iterator, which allows us to treat a hierarchy of containers as a single, flattened sequence.
Problem
Suppose we have a set of vectors containing integers, and we want to iterate over them as a single, contiguous list. A simple std::vector<std::vector<int>> might not suffice, as it requires navigating through each nested vector separately.
Custom flattening_iterator
To address this issue, we can create a custom iterator, flattening_iterator, that iterates through the nested containers sequentially. This iterator:
- Takes as its input an iterator to the outermost container.
- Keeps track of the current position within both the outermost and innermost containers.
- Advances the iterator by incrementally moving through the nested containers until it reaches the end or encounters an empty innermost container.
Implementation
The flattening_iterator is implemented as follows:
template <typename OuterIterator> class flattening_iterator { public: // ... iterator category, value type, etc. flattening_iterator(outer_iterator it) : outer_it_(it), outer_end_(it) { } flattening_iterator(outer_iterator it, outer_iterator end) : outer_it_(it), outer_end_(end) { advance_past_empty_inner_containers(); } // ... operators for comparison, dereferencing, and advancement private: void advance_past_empty_inner_containers() { // Advance until we find a non-empty innermost container while (outer_it_ != outer_end_ && inner_it_ == outer_it_->end()) { ++outer_it_; if (outer_it_ != outer_end_) inner_it_ = outer_it_->begin(); } } outer_iterator outer_it_; outer_iterator outer_end_; inner_iterator inner_it_; };
Usage
We can use the flattening_iterator to flatten nested containers as follows:
std::unordered_set<std::vector<int>> s; s.insert({}); s.insert({1,2,3,4,5}); s.insert({6,7,8}); s.insert({9,10,11,12}); // Create a flattening iterator and iterate over the flattened set for (auto it = flatten(s.begin()); it != s.end(); ++it) { std::cout << *it << endl; }
This will output the flattened list of all integers in the nested containers:
1 2 3 4 5 6 7 8 9 10 11 12
Conclusion
The flattening_iterator provides a simple and effective way to iterate over nested containers as a single, flattened sequence. This simplifies code and eliminates the need for complex nested loop structures or manual navigation through the nested hierarchy.
The above is the detailed content of How Can a Custom Iterator Simplify Iterating Through Nested Containers?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
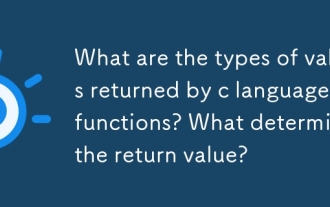
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
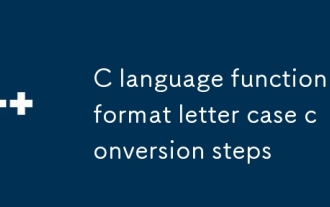
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
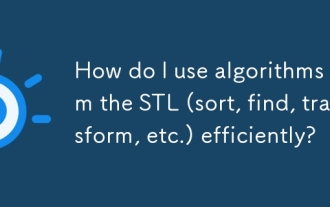
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
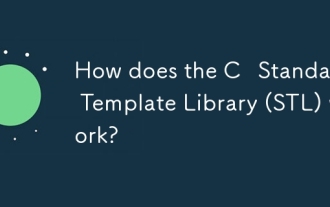
How does the C Standard Template Library (STL) work?
