


How to Avoid the \'Can\'t Marshal interface {} as a BSON document\' Error in Go?
How to Construct and Pass BSON Documents in Go Lang?
Introduction
MongoDB interacts with documents represented in Binary JSON (BSON) format. In Go, constructing and passing BSON documents for insertion into MongoDB can be challenging. This article will provide a solution to the common error encountered during this process.
The Error: Interface{} Cannot Marshal as BSON Document
When passing a BSON document to a function parameter defined as interface{}, an error may occur: "Can't marshal interface {} as a BSON document." This error indicates that Go cannot automatically marshal an arbitrary interface as a BSON document.
Solution: Use a Custom Struct
To resolve this issue, avoid using interface{} and instead define a custom struct to represent the BSON document. For example, consider the following BSON document:
{ "_id": "53439d6b89e4d7ca240668e5", "balanceamount": 3, "type": "reg", "authentication": { "authmode": "10", "authval": "sd", "recovery": { "mobile": "sdfsd", "email": "email@protected.com" } }, "stamps": { "in": "x", "up": "y" } }
In Go, the corresponding custom struct would be:
type Account struct { Id bson.ObjectId `bson:"_id"` BalanceAmount int // Other fields... }
Pass the Custom Struct to Insert Function
Now, in the dbEngine.go file, modify the Insert function to accept the custom struct as an argument:
func Insert(document *Account) { // Connect to MongoDB and insert the document }
Usage in the Application
To use this function, create an instance of the Account struct, populate its fields, and pass it to the Insert function:
acc := Account{ Id: bson.NewObjectId(), BalanceAmount: 3, // Other fields... } dbEngine.Insert(&acc)
Conclusion
By creating a custom struct to represent the BSON document and passing it to a function that accepts the specific struct type, the error can be avoided, and BSON documents can be constructed and passed seamlessly.
The above is the detailed content of How to Avoid the \'Can\'t Marshal interface {} as a BSON document\' Error in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










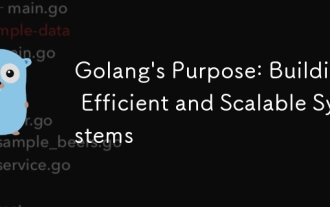
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
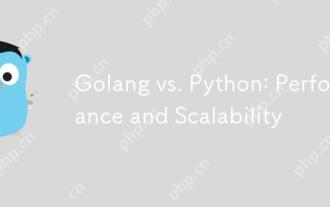
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
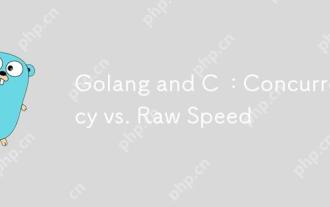
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
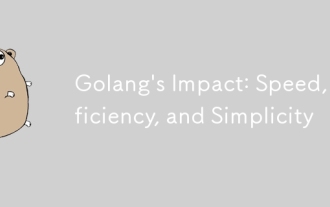
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
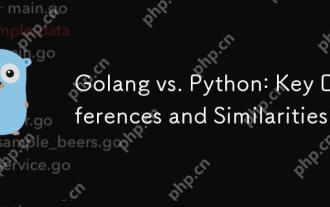
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
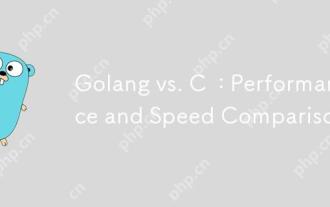
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
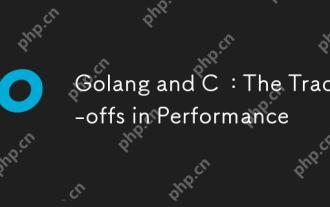
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
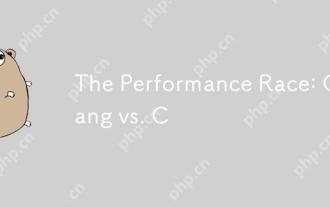
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
