How to Convert Java Objects to JSON using Jackson?
Converting Java Objects to JSON Using Jackson
Object Structure
To achieve your desired JSON output, your classes are structured correctly. ValueData represents the main object containing a list of ValueItems. Each ValueItems object represents an entry in the information array.
Object Mapping and JSON Conversion
To convert the ValueData object to JSON, you need to use the Jackson library:
- Add the following dependency to your project's pom.xml:
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.13.3</version> </dependency>
- In your main method, use Jackson to convert the object:
import com.fasterxml.jackson.databind.ObjectMapper; import com.fasterxml.jackson.databind.ObjectWriter; public static void main(String[] args) throws Exception { // Create Java object ValueData valueData = ... ; // Create and initialize the ValueData object ObjectWriter ow = new ObjectMapper().writer().withDefaultPrettyPrinter(); String json = ow.writeValueAsString(valueData); System.out.println(json); }
JSON Output
The writeValueAsString method generates the JSON string in the desired format:
{ "information": [{ "timestamp": "xxxx", "feature": "xxxx", "ean": 1234, "data": "xxxx" }, { "timestamp": "yyy", "feature": "yyy", "ean": 12345, "data": "yyy" }] }
This output matches the desired JSON format specified in your question.
The above is the detailed content of How to Convert Java Objects to JSON using Jackson?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










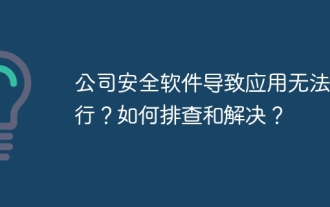
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
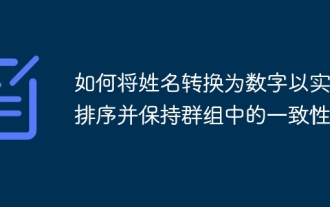
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
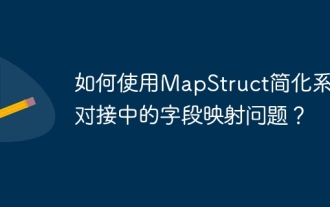
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
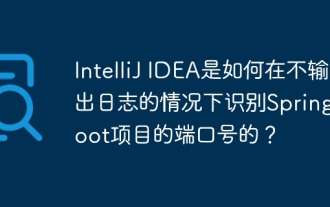
Start Spring using IntelliJIDEAUltimate version...
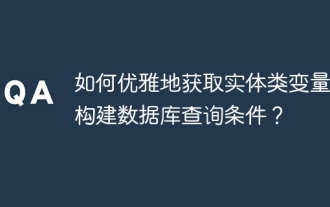
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
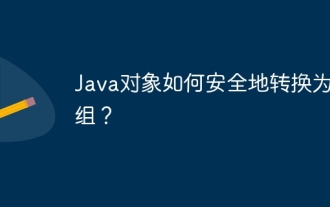
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
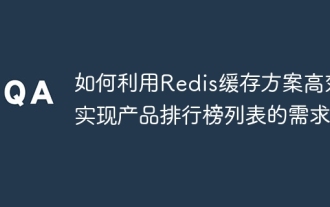
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
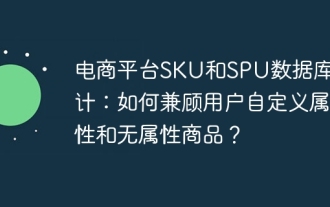
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
