How Can I Load Classpath Resources Using a Custom URL Protocol?
Using Classpath Protocol to Load Resources
Problem Statement:
Loading resources from the classpath using a URL protocol that does not require specifying the specific JAR file or class folder.
Solution:
Implementing a URL Stream Handler:
To create a protocol that loads resources from the classpath, implement a custom URLStreamHandler. This handler will open connections to URLs using the "classpath" protocol.
<br>public class Handler extends URLStreamHandler {</p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">private final ClassLoader classLoader; public Handler(ClassLoader classLoader) { this.classLoader = classLoader; } @Override protected URLConnection openConnection(URL u) throws IOException { final URL resourceUrl = classLoader.getResource(u.getPath()); return resourceUrl.openConnection(); }
}
Usage:
Use the custom handler to specify the classpath protocol in the URL when loading resources.
<br>new URL("classpath:org/my/package/resource.extension").openConnection();<br>
Handling Launch Issues:
Manual Code Handler Specification:
If possible, manually specify the custom handler when creating the URL.
<br>new URL(null, "classpath:some/package/resource.extension", new org.my.protocols.classpath.Handler(ClassLoader.getSystemClassLoader()))<br>
JVM Handler Registration:
Register a URLStreamHandlerFactory with the JVM to handle all URLs using the classpath protocol.
<br>URL.setURLStreamHandlerFactory(new ConfigurableStreamHandlerFactory("classpath", new Handler(ClassLoader.getSystemClassLoader())));<br>
Caveat:
JVM handler registration can only be called once per JVM, so be cautious when using it in environments where multiple handlers may conflict.
The above is the detailed content of How Can I Load Classpath Resources Using a Custom URL Protocol?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










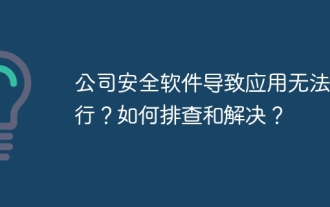
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
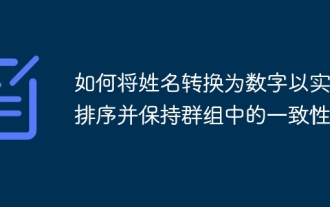
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
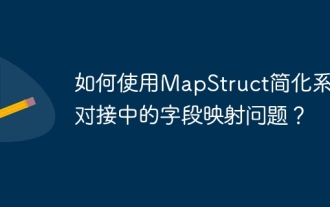
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
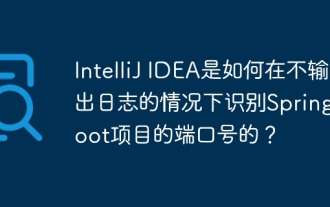
Start Spring using IntelliJIDEAUltimate version...
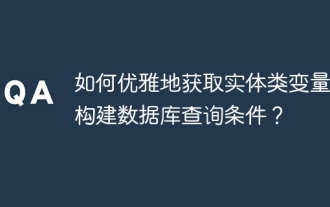
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
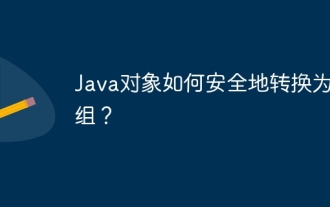
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
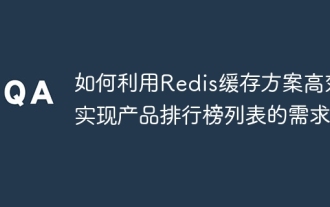
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
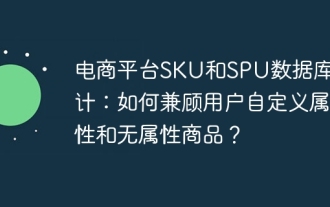
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
