


How Can I Migrate My Legacy PHP Code from mysql_* Functions to PDO for Enhanced Security and Stability?
Nov 26, 2024 am 09:13 AMRewriting Legacy PHP Code with Deprecated mysql_* Functions Using PDO
Introduction
With the increasing security concerns and the need for more reliable database interactions, the mysql_* functions have become obsolete in PHP. This transition necessitates rewriting old code to use the more secure and stable prepared statements and PDO. Here's a comprehensive guide to help you navigate this process:
Constructor and Destructor
The __construct and __destruct methods were used for setting up and closing database connections. However, PDO simplifies this process through its constructor and built-in connection management.
Connect Function
The connect function is replaced by the PDO constructor, which manages database connections and error handling internally.
Select Database Function
The selectDb function, which was responsible for selecting a specific database, is no longer necessary with PDO. This functionality is now embedded in the connection string itself.
Simplified Code
Here's a comparison of the old and new code:
Old Code
$db = new dbConn('127.0.0.1', 'root', 'pass', 'people', 'animals'); $db->connect(); $db->selectDb("people");
New Code (PDO)
$db = new PDO('mysql:host=127.0.0.1;dbname=people;charset=UTF-8', 'root', 'pass');
Potential Extensions
You can extend the functionality of PDO by creating your own custom database class that extends from PDO:
class DB extends PDO { ... custom code }
Additional Resources
For more detailed information, refer to the following resources:
- PHP/MySQL Table with Hyperlinks: https://eoneil.org/articles/php/mysql/hyperlinkTable.php
- PDO Tutorial for MySQL Developers: https://www.php.net/manual/en/book.pdo.php
The above is the detailed content of How Can I Migrate My Legacy PHP Code from mysql_* Functions to PDO for Enhanced Security and Stability?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
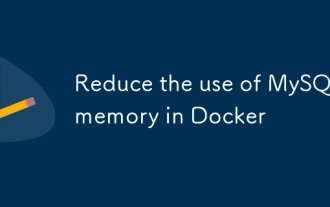
Reduce the use of MySQL memory in Docker
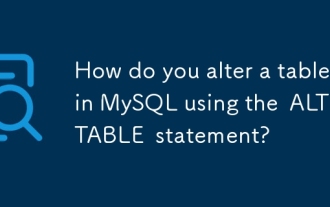
How do you alter a table in MySQL using the ALTER TABLE statement?
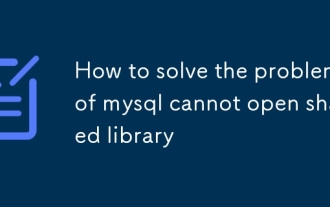
How to solve the problem of mysql cannot open shared library
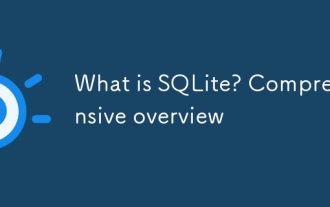
What is SQLite? Comprehensive overview
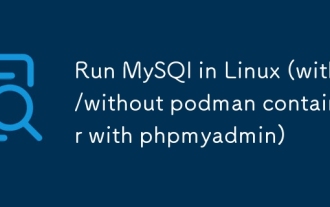
Run MySQl in Linux (with/without podman container with phpmyadmin)
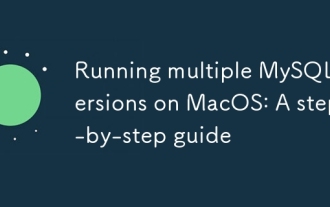
Running multiple MySQL versions on MacOS: A step-by-step guide
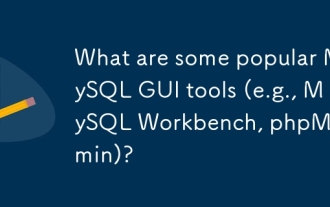
What are some popular MySQL GUI tools (e.g., MySQL Workbench, phpMyAdmin)?
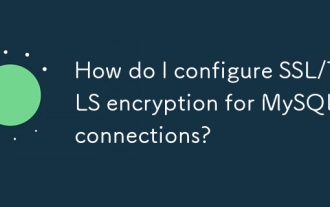
How do I configure SSL/TLS encryption for MySQL connections?
