How to Run Selenium Headless Using Xvfb on Amazon EC2?
Running Selenium Headless Using Xvfb on Amazon EC2
You're attempting to run Selenium on an Amazon EC2 instance where no GUI is present. After installing the necessary packages and initiating Xvfb, you encounter the error "Error: cannot open display: :0" when executing your code. This error stems from the lack of a graphical display on the EC2 instance.
To resolve this issue, consider utilizing PyVirtualDisplay or xvfbwrapper, which enable you to launch Selenium in a virtual display environment. These modules create a headless X server, allowing WebDriver tests to run without a physical GUI.
PyVirtualDisplay Method
from pyvirtualdisplay import Display from selenium import webdriver display = Display(visible=0, size=(800, 600)) display.start() browser = webdriver.Firefox() browser.get('http://www.google.com') print browser.title browser.quit() display.stop()
This code snippet employs PyVirtualDisplay to initiate a headless virtual display environment. Within this environment, the Firefox browser is launched and navigates to a specified URL. After accessing the page title, the browser is terminated, and the virtual display is closed.
Xvfbwrapper Method
from xvfbwrapper import Xvfb vdisplay.start() browser = webdriver.Firefox() browser.get('http://www.google.com') print browser.title browser.quit() vdisplay.stop()
This code employs Xvfbwrapper to start a virtual display. Similarly to the PyVirtualDisplay example, a Firefox browser is launched within the virtual display, pages are visited, and the browser and display are terminated.
Context Manager Method
from xvfbwrapper import Xvfb with Xvfb() as xvfb: browser = webdriver.Firefox() browser.get('http://www.google.com') print browser.title browser.quit()
This method uses a context manager to start and stop the virtual display automatically. Within the context block, a Firefox browser is launched, pages are visited, and the browser is closed.
By adopting these methods, you can seamlessly run Selenium headless tests on Amazon EC2 instances without a GUI, enabling automated testing and efficient deployment.
The above is the detailed content of How to Run Selenium Headless Using Xvfb on Amazon EC2?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










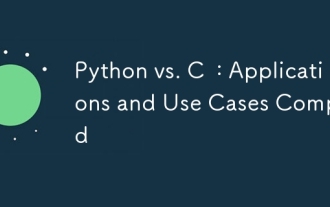
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
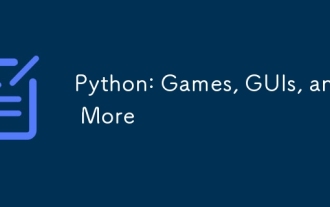
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
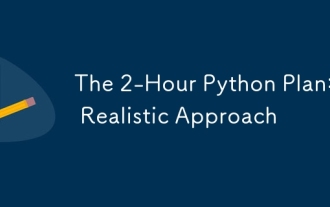
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
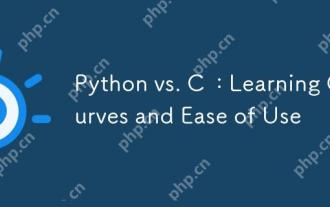
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
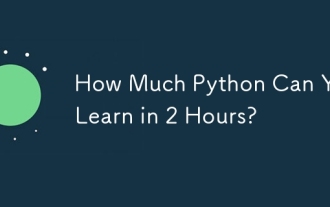
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
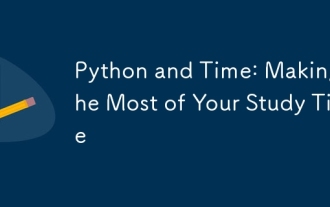
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
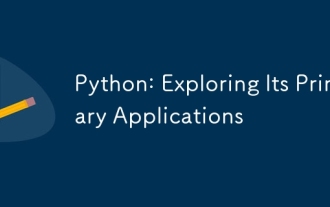
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
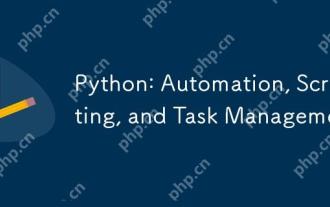
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
