How to Efficiently Iterate Through Nested JSON in Go?
Looping/Iterating Over Second Level Nested JSON in Go Lang
Consider the scenario where you encounter a nested JSON structure like the one depicted below:
{ "outterJSON": { "innerJSON1": { "value1": 10, "value2": 22, "InnerInnerArray": [ "test1" , "test2"], "InnerInnerJSONArray": [ {"fld1" : "val1"} , {"fld2" : "val2"} ] }, "InnerJSON2":"NoneValue" } }
The task is to effectively iterate through this structure and retrieve all key-value pairs as strings for further processing. Unfortunately, manually defining a struct for such a dynamic JSON input is not feasible.
Efficient Iteration Approach
To navigate this challenge efficiently, a recursive approach is employed:
func parseMap(m map[string]interface{}) { for key, val := range m { // Check the type of the value switch concreteVal := val.(type) { case map[string]interface{}: // If it's a nested map, recursively call the function parseMap(val.(map[string]interface{})) case []interface{}: // If it's a nested array, call the function to parse the array parseArray(val.([]interface{})) default: // For all other types, print the key and value as a string fmt.Println(key, ":", concreteVal) } } }
This recursive function parseMap examines the type of each value in the map. If the value is itself a map, it recursively calls parseMap to traverse that nested map. If the value is an array, it calls parseArray to iterate over it. For all other types (such as strings, numbers, etc.), it simply prints the key and value as a string.
Demo
Consider the example JSON input provided earlier. Running the code below will produce the following output:
func parseArray(a []interface{}) { for i, val := range a { // Check the type of the value switch concreteVal := val.(type) { case map[string]interface{}: // If it's a nested map, recursively call the function parseMap(val.(map[string]interface{})) case []interface{}: // If it's a nested array, call the function to parse the array parseArray(val.([]interface{})) default: // For all other types, print the index and value as a string fmt.Println("Index:", i, ":", concreteVal) } } } const input = ` { "outterJSON": { "innerJSON1": { "value1": 10, "value2": 22, "InnerInnerArray": [ "test1" , "test2"], "InnerInnerJSONArray": [{"fld1" : "val1"} , {"fld2" : "val2"}] }, "InnerJSON2":"NoneValue" } } `
Output:
//outterJSON //innerJSON1 //InnerInnerJSONArray //Index: 0 //fld1 : val1 //Index: 1 //fld2 : val2 //value1 : 10 //value2 : 22 //InnerInnerArray //Index 0 : test1 //Index 1 : test2 //InnerJSON2 : NoneValue
This approach effectively captures all key-value pairs within the nested JSON, making it suitable for processing and extraction tasks in Go lang.
The above is the detailed content of How to Efficiently Iterate Through Nested JSON in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










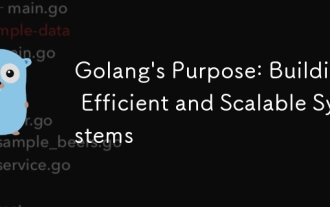
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
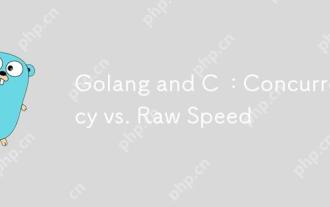
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
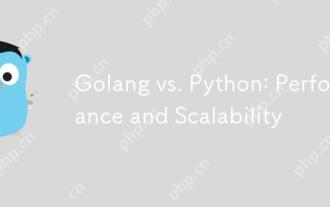
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
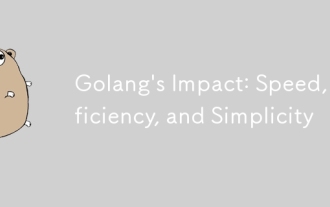
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
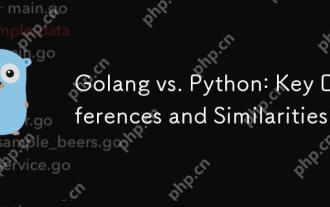
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
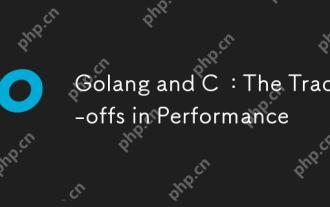
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
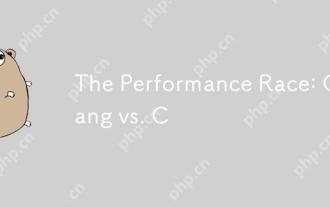
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
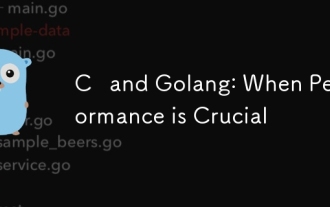
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
