


How Can Pandas Efficiently Convert String Dates to DateTime Objects and Filter by Date Range?
Converting String Formats to Datetime Format in Pandas
Pandas provides a convenient way to convert string values representing dates and times into datetime objects. The pd.to_datetime() function can handle a variety of input string formats, automatically detecting the correct format based on the value's contents.
Consider the following column of string values representing dates:
I_DATE 28-03-2012 2:15:00 PM 28-03-2012 2:17:28 PM 28-03-2012 2:50:50 PM
To convert I_DATE to datetime format, simply use pd.to_datetime(df['I_DATE']). Since the format is straightforward, pandas will automatically identify it.
In [51]: pd.to_datetime(df['I_DATE']) Out[51]: 0 2012-03-28 14:15:00 1 2012-03-28 14:17:28 2 2012-03-28 14:50:50 Name: I_DATE, dtype: datetime64[ns]
You can also access specific components of the datetime object using the dt accessor:
In [54]: df['I_DATE'].dt.date Out[54]: 0 2012-03-28 1 2012-03-28 2 2012-03-28 dtype: object In [56]: df['I_DATE'].dt.time Out[56]: 0 14:15:00 1 14:17:28 2 14:50:50 dtype: object
Filtering Data Based on Date Ranges
Once your data is in datetime format, you can easily filter based on date ranges. For example, to filter the df DataFrame for rows where I_DATE falls within a specific range, you can use:
df[(df['I_DATE'] > '2015-02-04') & (df['I_DATE'] < '2015-02-10')] Out[59]: date 35 2015-02-05 36 2015-02-06 37 2015-02-07 38 2015-02-08 39 2015-02-09
The above is the detailed content of How Can Pandas Efficiently Convert String Dates to DateTime Objects and Filter by Date Range?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










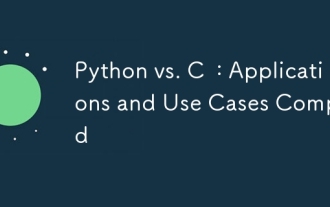
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
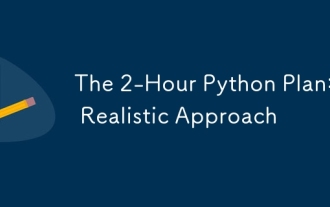
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
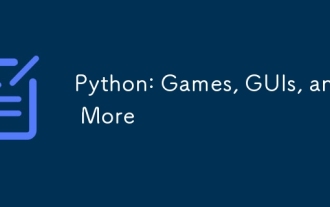
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
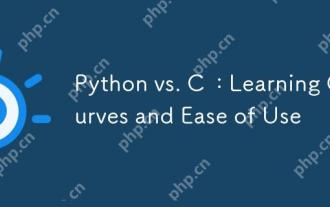
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
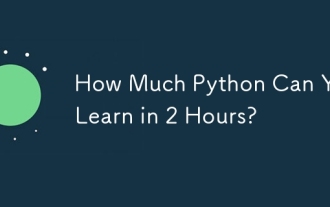
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
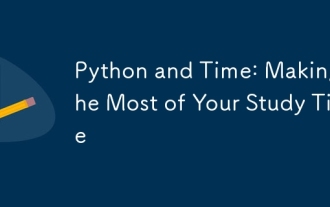
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
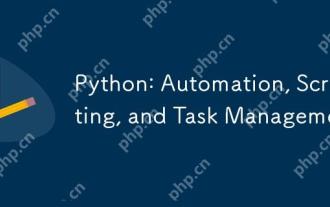
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
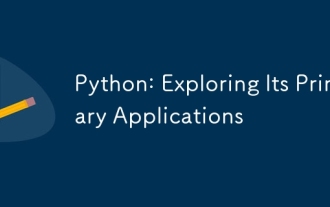
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
