


How Can Parameterization Improve Dynamic Unit Testing in Python?
Dynamic Unit Testing in Python with Parameterization
Unit testing plays a crucial role in ensuring the accuracy and reliability of code. When dealing with multiple test cases, creating individual tests for each can become tedious and time-consuming. Parameterization in Python offers an elegant solution to generate dynamic unit tests on the fly.
What is Parameterization?
Parameterization is a testing technique that involves passing different sets of data as parameters to a test function. This allows for the creation of multiple test cases based on a single test method, eliminating the need for repetitive code.
Implementing Parameterization in Python
Python provides several libraries to support parametrization, such as pytest and parameterized. Here's an example using the parameterized library:
import unittest from parameterized import parameterized class TestSequence(unittest.TestCase): @parameterized.expand([ ["foo", "a", "a"], ["bar", "a", "b"], ["lee", "b", "b"] ]) def test_sequence(self, name, a, b): self.assertEqual(a, b)
This code defines a parameterized test method that will automatically generate three test cases based on the provided data. Each test case will use the values from the corresponding list in the expand() decorator.
Benefits of Parameterization
- Reduced Code Duplication: Eliminates the need for manual creation of multiple test methods, reducing maintenance overhead.
- Increased Test Coverage: Allows for testing different scenarios with a single test method, improving test coverage.
- Improved Readability: Makes test code more concise and easier to understand by separating test data from test logic.
Conclusion
Parameterization in Python is a powerful technique for generating dynamic unit tests. It simplifies test code, reduces duplication, and improves test coverage by allowing for multiple test cases to be defined using a single test method. This makes it an essential tool for efficient and effective software testing.
The above is the detailed content of How Can Parameterization Improve Dynamic Unit Testing in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










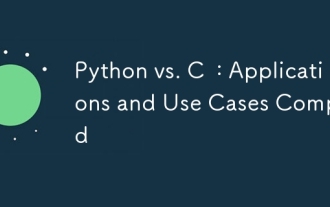
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
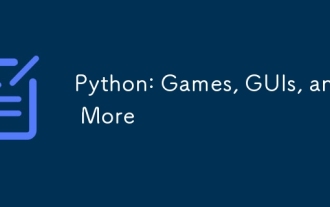
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
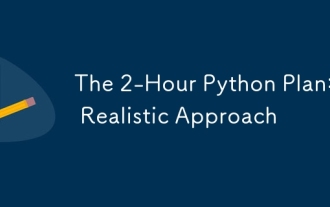
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
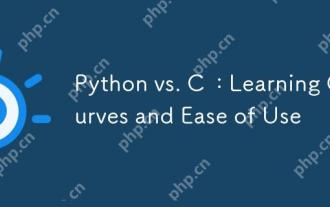
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
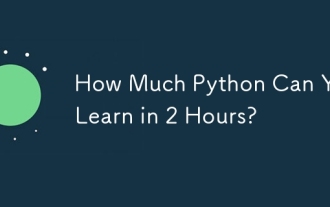
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
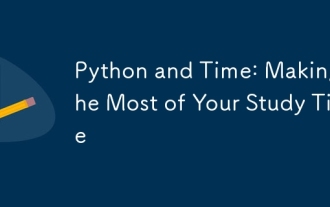
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
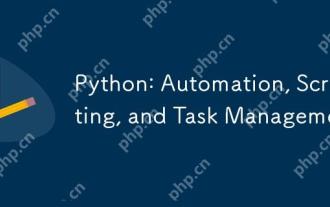
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
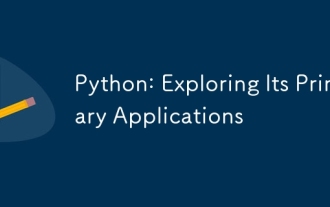
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
