


When should I use `await` in Python 3.5 asynchronous programming, and what are its limitations?
When to Employ and the Limitations of await in Python 3.5
Asynchrony in Python 3.5 is primarily facilitated through the asyncio library and the async/await syntax. Understanding when and where to leverage these constructs can be crucial for optimizing the performance of your asynchronous applications.
The decision to use await should hinge on the nature of your code. By default, your code will run synchronously. To introduce asynchrony, you can define functions using async def and invoke them with await. However, it's important to determine whether synchronous or asynchronous code is more appropriate for the task at hand.
As a general rule of thumb, it's beneficial to use await when dealing with I/O operations. I/O operations, such as network requests or database calls, are often inherently asynchronous and can be significantly accelerated by delegating them to the event loop.
For example, consider the following synchronous code:
download(url1) # takes 5 seconds download(url2) # takes 5 seconds # Total time: 10 seconds
Using asyncio and await, the same code can be rewritten asynchronously, reducing the total execution time to the time taken for the longer operation:
await asyncio.gather( async_download(url1), # takes 5 seconds async_download(url2), # takes 5 seconds ) # Total time: only 5 seconds (plus minimal asyncio overhead)
It's also important to note that any asynchronous function can freely utilize synchronous code if necessary. However, casting synchronous code to asynchronous without a valid reason should be avoided, as it does not inherently introduce any benefits.
One crucial consideration with asynchronous code is the potential for long-running synchronous operations to freeze the entire program. Any synchronous operation that exceeds a certain threshold (e.g., 50 milliseconds) can block any concurrent asynchronous tasks.
To mitigate this issue, you can outsource such operations to a separate process and await their results:
executor = ProcessPoolExecutor(2) async def extract_links(url): ... # If search_in_very_big_file() is a long synchronous operation, offload it to a separate process links_found = await loop.run_in_executor(executor, search_in_very_big_file, links)
Finally, it's worth noting that I/O-bound synchronous functions can be integrated into asynchronous code using run_in_executor() along with a ThreadPoolExecutor to minimize the overhead associated with multiprocessing.
The above is the detailed content of When should I use `await` in Python 3.5 asynchronous programming, and what are its limitations?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




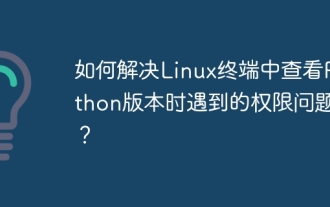
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
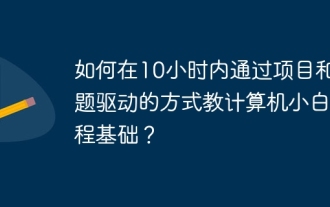
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
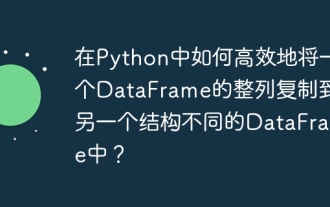
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
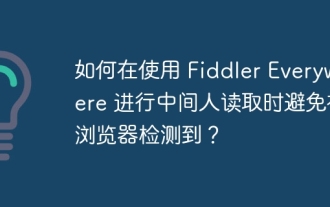
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
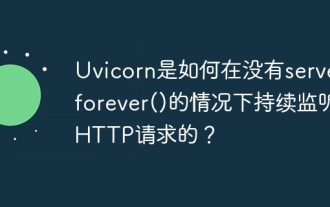
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
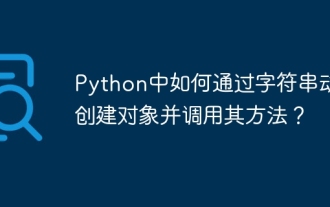
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
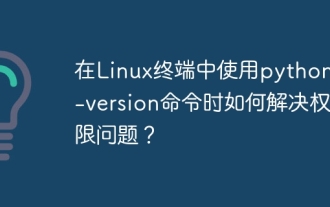
Using python in Linux terminal...
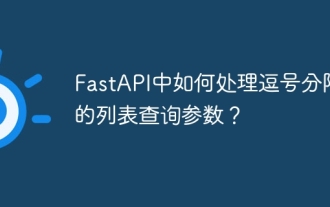
Fastapi ...
