


Why Doesn\'t Java Throw a NullPointerException When Concatenating Null Strings?
Nov 26, 2024 pm 06:56 PMWhy Null Strings Concatenate in Java
In Java, it is possible to concatenate null strings without encountering a NullPointerException. This behavior occurs because of the way the Java Virtual Machine (JVM) handles null references:
Handling Null References
According to the Java Language Specification (JLS), if a reference is null, it is converted to the string "null" when performing string concatenation. This conversion is performed automatically by the JVM. As a result, the expression s "hello" does not raise an exception but instead evaluates to the string "nullhello", even if s is initially null.
Implementation Details
The JVM handles null references during concatenation by generating bytecode that uses the StringBuilder class. The compiler effectively translates the concatenation operation into code that initializes a StringBuilder object, appends the non-null string ("hello" in this case), and converts the result to a String object.
The code below shows the equivalent bytecode that the compiler generates:
String s = null; s = new StringBuilder(String.valueOf(s)).append("hello").toString();
This code uses the StringBuilder class to handle the null reference safely and concatenate the strings.
Optimization Considerations
For performance reasons, the JVM can optimize repeated string concatenations by using the StringBuffer class or a similar technique. This optimization avoids creating unnecessary intermediate String objects during evaluation. However, the exact implementation of this optimization may vary across different compilers.
The above is the detailed content of Why Doesn\'t Java Throw a NullPointerException When Concatenating Null Strings?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
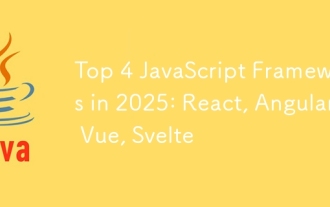
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
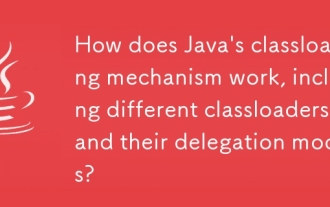
How does Java's classloading mechanism work, including different classloaders and their delegation models?
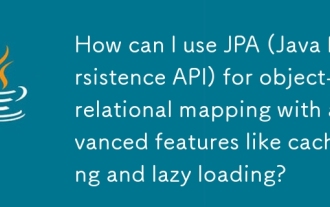
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
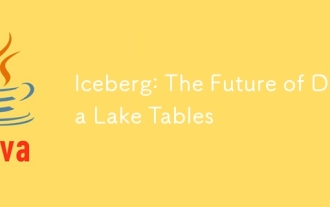
Iceberg: The Future of Data Lake Tables
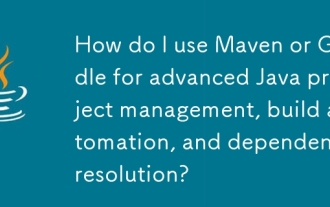
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
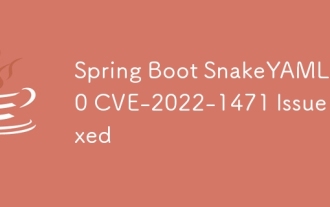
Spring Boot SnakeYAML 2.0 CVE-2022-1471 Issue Fixed
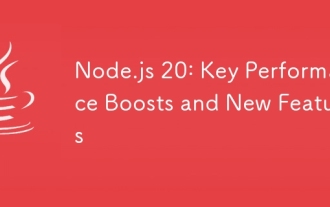
Node.js 20: Key Performance Boosts and New Features
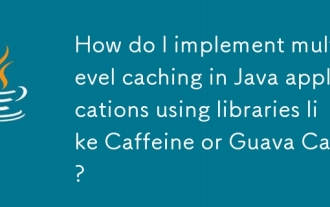
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
