


Why Do Loop-Based Click Handlers in JavaScript Sometimes Display Unexpected Values?
Handling Click Events in a Loop: Understanding Closure Pitfalls
When assigning click handlers to multiple elements with a loop, it's essential to be aware of JavaScript's closure mechanism. A common mistake is to create closures in a loop without using callback functions. This can lead to unexpected behavior.
In the code snippet provided:
$(document).ready(function(){ for(var i = 0; i < 20; i++) { $('#question' + i).click( function(){ alert('you clicked ' + i); }); } });
The expected behavior is that clicking on #mydiv3 would display "you clicked 3." However, the code is incorrectly using the i variable, which is a global variable in the loop. As a result, the i variable holds the final value of 20, causing an incorrect alert message.
The correct way to assign click handlers in a loop is to use callback functions. These functions can create a new scope for the i variable, ensuring that each iteration of the loop has its own instance of i.
function createCallback(i){ return function(){ alert('you clicked ' + i); } } $(document).ready(function(){ for(var i = 0; i < 20; i++) { $('#question' + i).click(createCallback(i)); } });
Another modern solution, if using ES6, is to utilize the let keyword to create a local variable for each iteration of the loop:
for(let i = 0; i < 20; i++) { $('#question' + i).click( function(){ alert('you clicked ' + i); }); }
This approach is cleaner and easier to understand. It ensures that each click handler has its own i variable, eliminating closure pitfalls and ensuring correct behavior when handling click events in a loop.
The above is the detailed content of Why Do Loop-Based Click Handlers in JavaScript Sometimes Display Unexpected Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


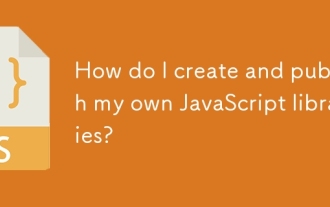
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
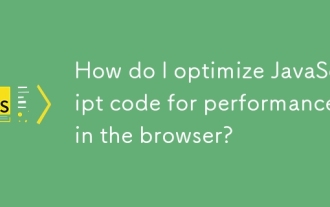
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
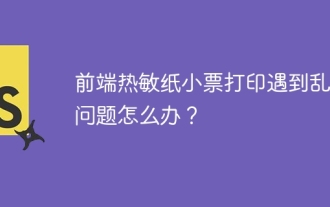
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
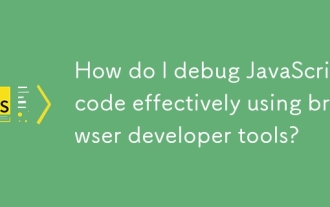
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
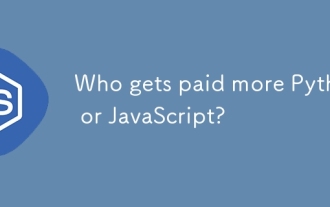
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
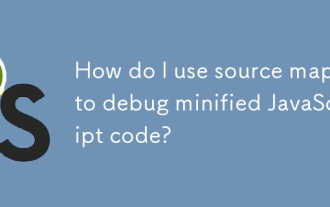
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
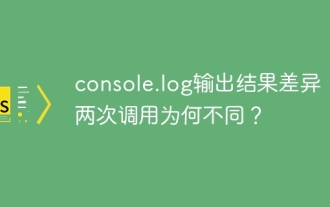
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
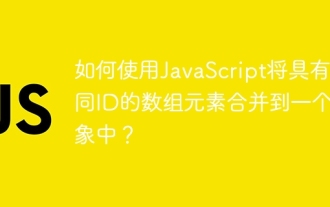
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
