


How Can I Create a PHP Function to Filter a Two-Dimensional Array Based on Value?
Creating a Function for Value-Based Filtering of Two-Dimensional Arrays
Filtering specific data from multi-dimensional arrays is a common task in programming. This task can be accomplished using various techniques, including PHP's array_filter function.
Function Creation
To create a function that filters a two-dimensional array by value, follow these steps:
- Define the function with the input array as a parameter.
- Utilize array_filter with an anonymous or callback function as the second parameter.
- Within the callback function, use conditional logic to specify the filtering criteria.
Example Implementation
Consider the following array:
$arr = [ [ 'interval' => '2014-10-26', 'leads' => 0, 'name' => 'CarEnquiry', 'status' => 'NEW', 'appointment' => 0 ], [ 'interval' => '2014-10-26', 'leads' => 0, 'name' => 'CarEnquiry', 'status' => 'CALL1', 'appointment' => 0 ], [ 'interval' => '2014-10-26', 'leads' => 0, 'name' => 'Finance', 'status' => 'CALL2', 'appointment' => 0 ], [ 'interval' => '2014-10-26', 'leads' => 0, 'name' => 'Partex', 'status' => 'CALL3', 'appointment' => 0 ] ];
To filter this array for values containing 'CarEnquiry' in the 'name' key:
function filterArrayByName($arr) { return array_filter($arr, function($var) { return $var['name'] == 'CarEnquiry'; }); }
Customizable Filtering
To make the search value interchangeable, modify the callback function as follows:
function filterArrayByName($arr, $filterBy) { return array_filter($arr, function($var) use ($filterBy) { return $var['name'] == $filterBy; }); }
Now you can use the function to filter the array by any desired value in the 'name' key.
The above is the detailed content of How Can I Create a PHP Function to Filter a Two-Dimensional Array Based on Value?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










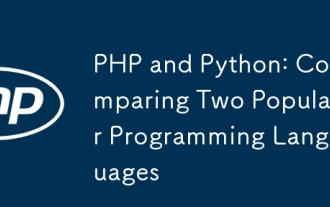
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
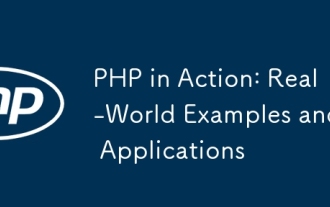
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
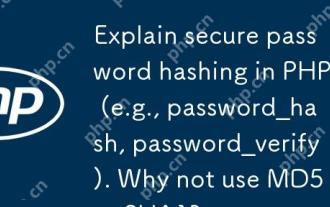
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
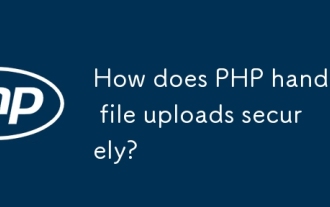
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
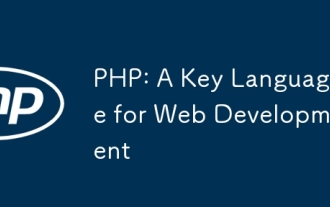
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
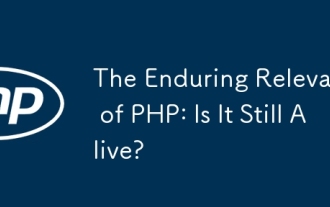
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
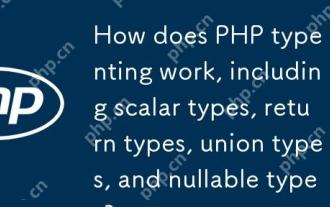
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
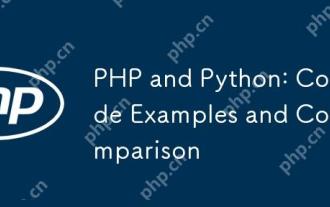
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
