Synchronization and Communication between Threads
Additional content:
Synchronization and Communication between Threads
Issue: Threads can interfere with each other when accessing shared data.
Solution:
Synchronized methods
synchronized void synchronizedMethod() { // Código sincronizado }
Synchronized blocks:
synchronized (this) { // Código sincronizado }
Example of Communication:
Communication between threads using wait(), notify() and notifyAll():
class SharedResource { private boolean flag = false; synchronized void produce() { while (flag) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println("Producing..."); flag = true; notify(); } synchronized void consume() { while (!flag) { try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println("Consuming..."); flag = false; notify(); } } public class ThreadCommunication { public static void main(String[] args) { SharedResource resource = new SharedResource(); Thread producer = new Thread(resource::produce); Thread consumer = new Thread(resource::consume); producer.start(); consumer.start(); } }
Conclusion
- Multi-threaded programming in Java allows you to create more efficient applications, especially on multicore systems.
- It is important to correctly manage access to shared resources using synchronization.
- The Thread class methods and the Runnable interface are powerful tools for working with multitasking.
The above is the detailed content of Synchronization and Communication between Threads. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










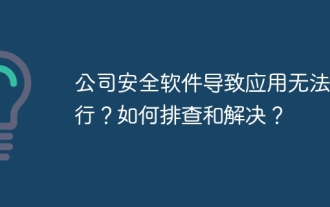
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
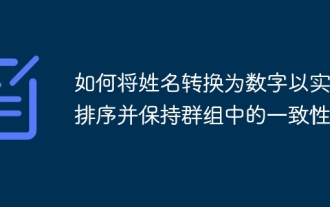
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
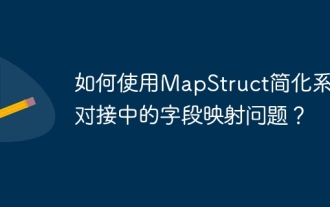
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
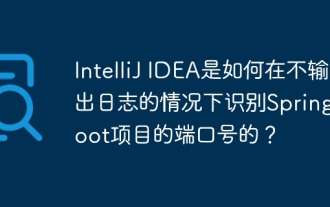
Start Spring using IntelliJIDEAUltimate version...
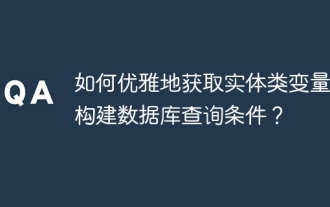
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
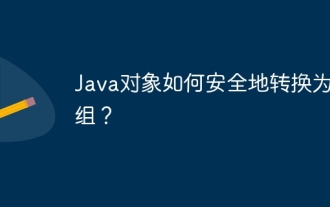
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
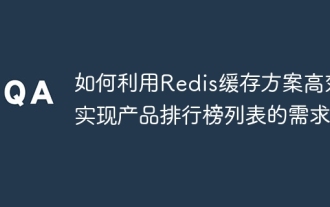
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
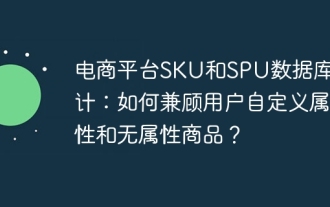
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
