


How Do You Determine the Maximum Nesting Depth of a PHP Array?
Nov 27, 2024 pm 12:17 PMDetermining Array Depth in PHP
Many PHP arrays contain embedded arrays, creating nested structures. Determining the maximum nesting depth of an array can provide insights into its complexity.
Sample Array:
$array = [ 'level1' => 'value1', 'level2' => [ 'level3' => [ 'level4' => 'value4', ], ], ];
Finding Array Depth Using Indentation:
One approach to calculating array depth is by leveraging print_r()'s output. This function provides a hierarchical representation of the array structure:
function array_depth($array) { $array_str = print_r($array, true); $lines = explode("\n", $array_str); $max_indentation = 1; foreach ($lines as $line) { $indentation = (strlen($line) - strlen(ltrim($line))) / 4; $max_indentation = max($max_indentation, $indentation); } return ceil(($max_indentation - 1) / 2) + 1; } echo array_depth($array); // Output: 4
This function calculates the maximum indentation level of the array. The formula ceil(($max_indentation - 1) / 2) 1 converts the indentation levels into an array depth.
The above is the detailed content of How Do You Determine the Maximum Nesting Depth of a PHP Array?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
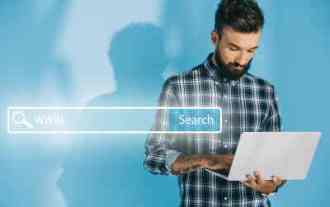
11 Best PHP URL Shortener Scripts (Free and Premium)
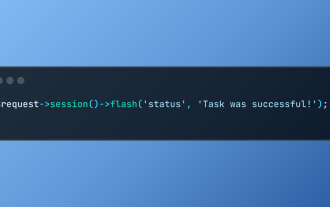
Working with Flash Session Data in Laravel
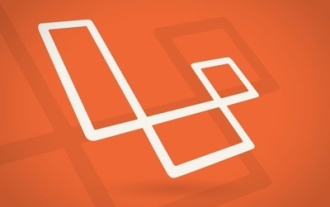
Build a React App With a Laravel Back End: Part 2, React
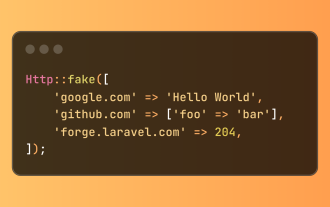
Simplified HTTP Response Mocking in Laravel Tests
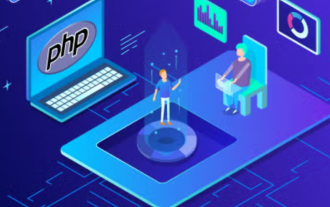
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
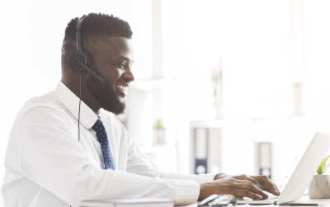
12 Best PHP Chat Scripts on CodeCanyon
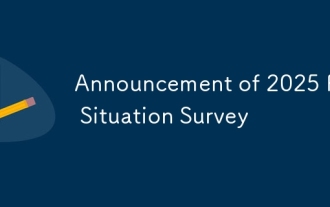
Announcement of 2025 PHP Situation Survey
